Create PDF Document with iText in Java
Many applications need to generate dynamic PDF documents. These documents vary from invoices, ebooks or vouchers etc. There are literally endless use-cases. This’ll be the first out of a series of tutorials regarding iText PDF document generation. And we start by showing how to create pdf documents using iText.
IText Dependency
In this example we used the latest version of iText. You can download the the iText library or add the following maven dependency to your project, and Maven’ll resolve the dependencies automatically.
<dependency>
<groupId>com.itextpdf</groupId>
<artifactId>itextpdf</artifactId>
<version>5.5.9</version>
</dependency>
There are different versions of iText. Up until version 2.1.7 all packages started with com.lowagie
. And these libraries where published under the LGPL/MPL licence. After version 5.x the packages started with com.itextpdf
and the licence changed to AGPL.
Create PDF Documents iText
- Create a document. The
com.itextpdf.text.Document
is the main class for PDF document generation. The first argument is the page size. The next arguments are left, right, top and bottom margins respectively. - Create a PdfWriter The
com.itextpdf.text.pdf.PdfWriter
is responsible for writing the content of the document to the output stream. The first argument is a reference to the document. The second argument is theFileOutputStream
in which the output will be written. - Open document. Once the document is opened, you can’t write any Header- or Meta-information anymore. You have to open the document before you can begin to add content to the body of the document.
- Add content. When the document is opened, you can start adding content.
- Close document. Closes the document. Once all the content has been written in the body, you have to close the body. After that nothing can be written to the body anymore.
package com.memorynotfound.pdf.itext;
import com.itextpdf.text.Document;
import com.itextpdf.text.DocumentException;
import com.itextpdf.text.PageSize;
import com.itextpdf.text.Paragraph;
import com.itextpdf.text.pdf.PdfWriter;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
public class CreatePdf {
public static void main(String... args) throws FileNotFoundException, DocumentException {
// 1. Create document
Document document = new Document(PageSize.A4, 50, 50, 50, 50);
// 2. Create PdfWriter
PdfWriter.getInstance(document, new FileOutputStream("result.pdf"));
// 3. Open document
document.open();
// 4. Add content
document.add(new Paragraph("Create Pdf Document with iText in Java"));
// 5. Close document
document.close();
}
}
Output
After the application executes successfully, the result.pdf document is created under the root of your application class-path.
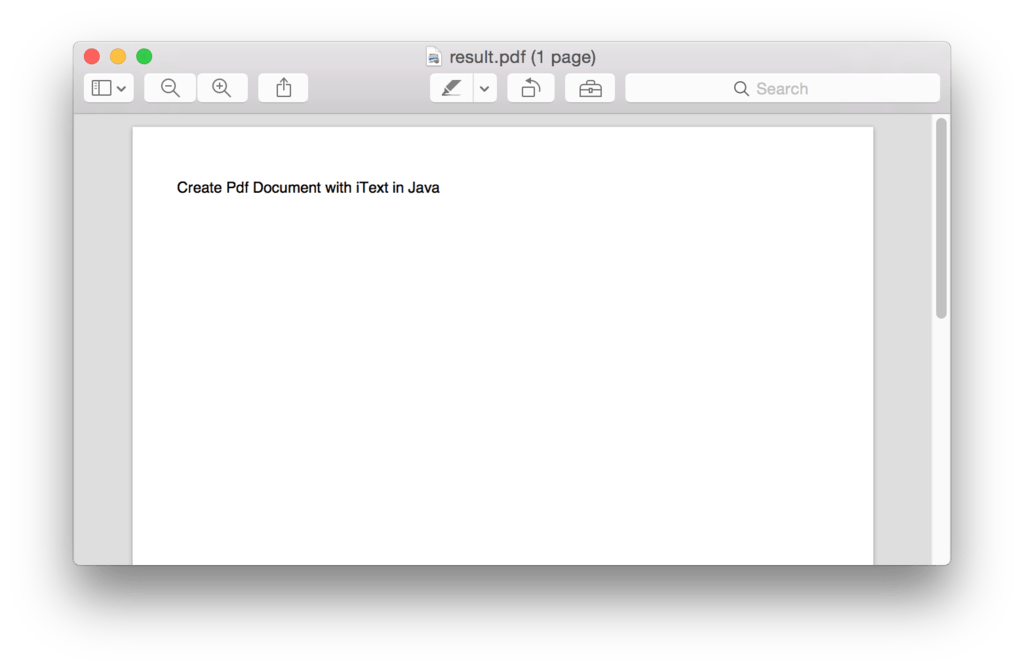