Chapter 2 Elementary Programming Liang Introduction to Programming
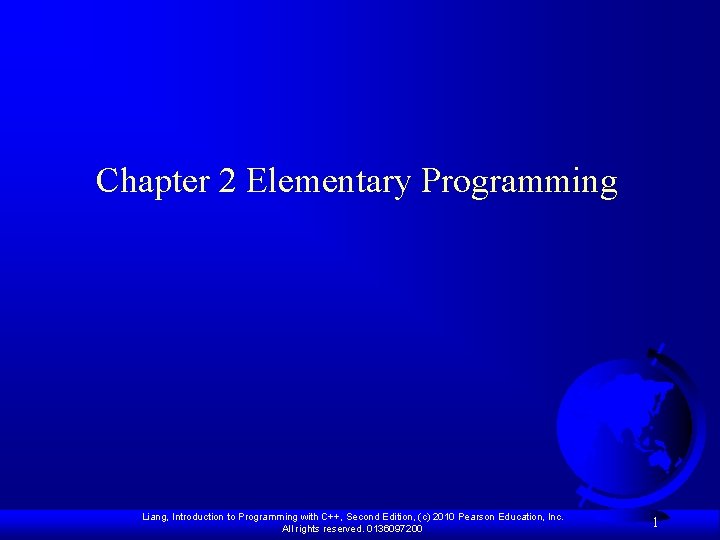
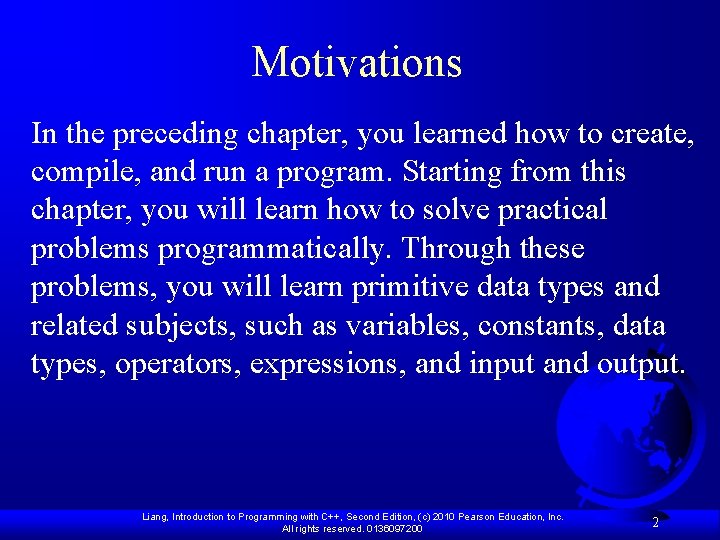
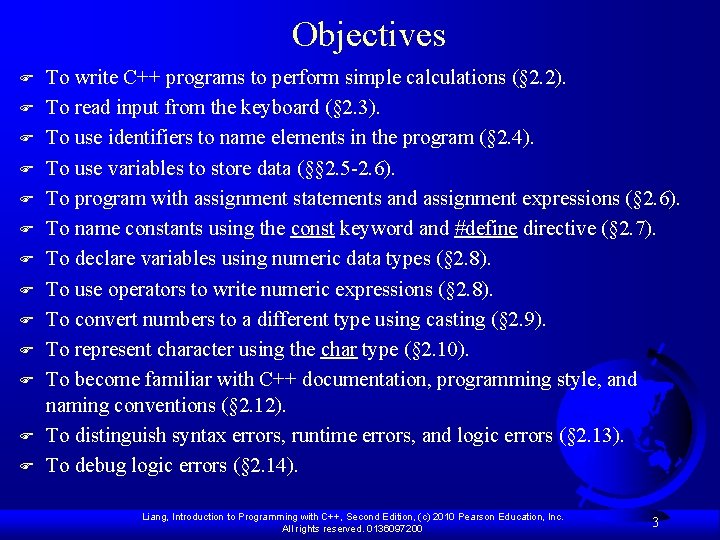
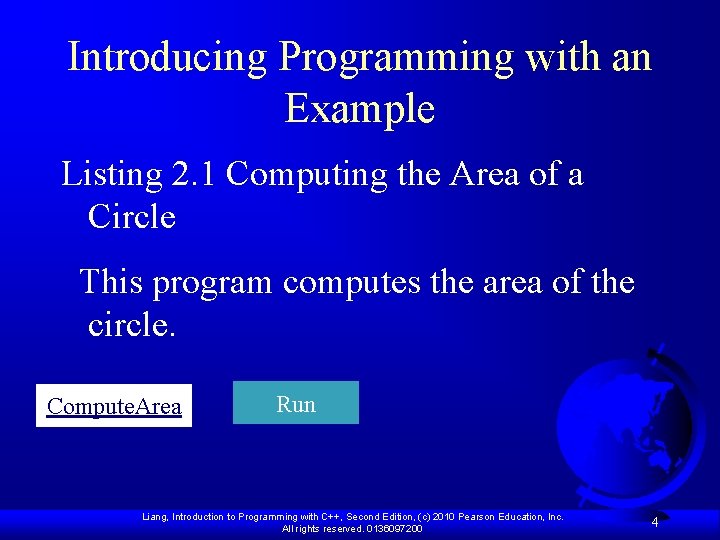
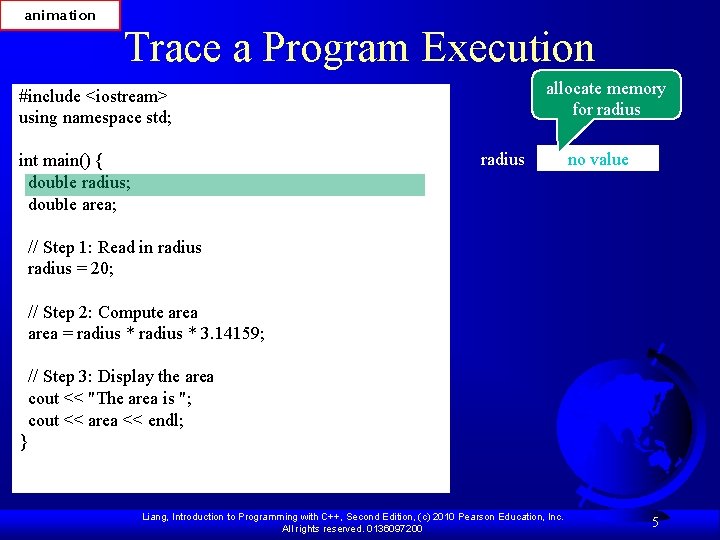
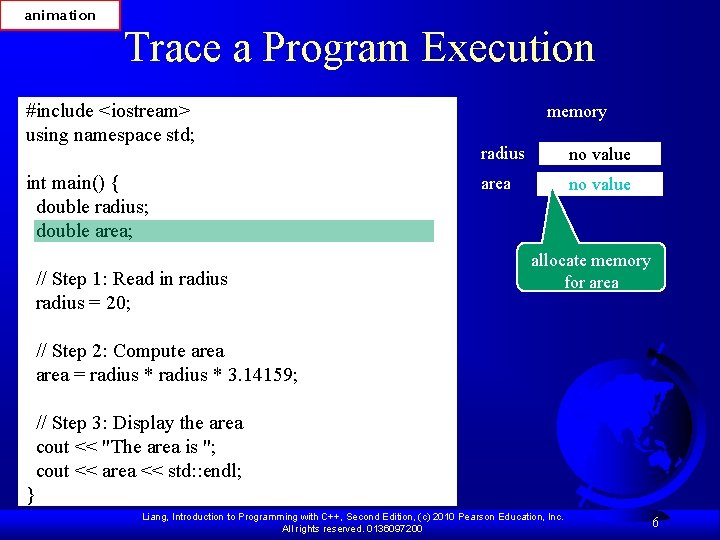
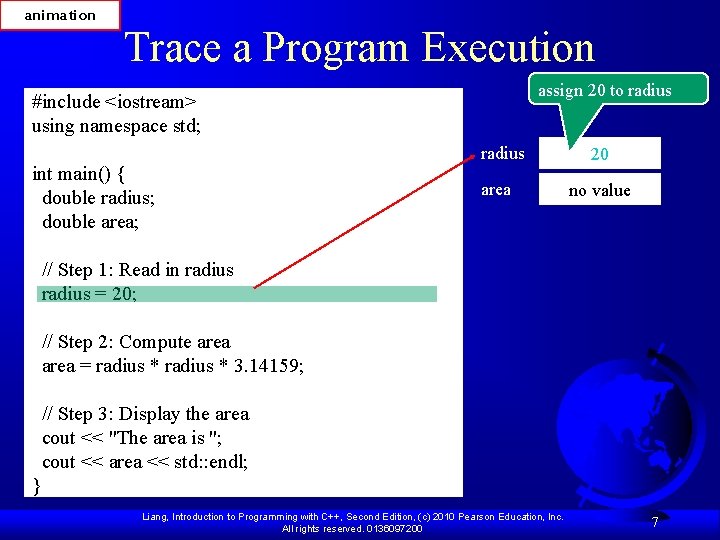
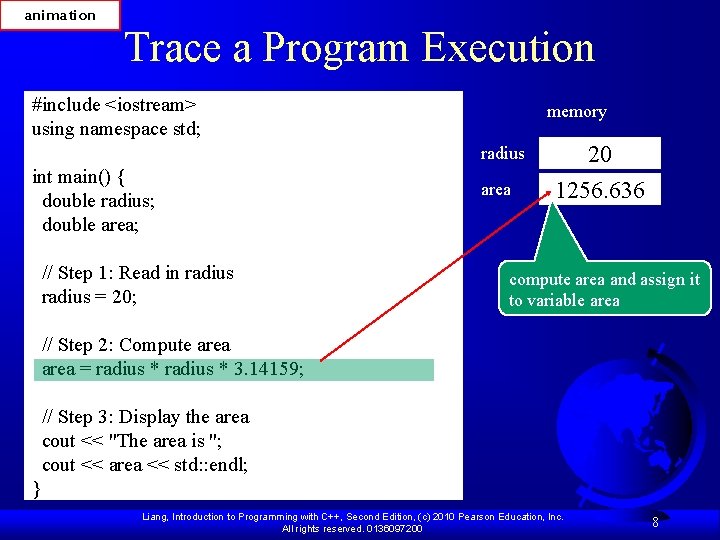
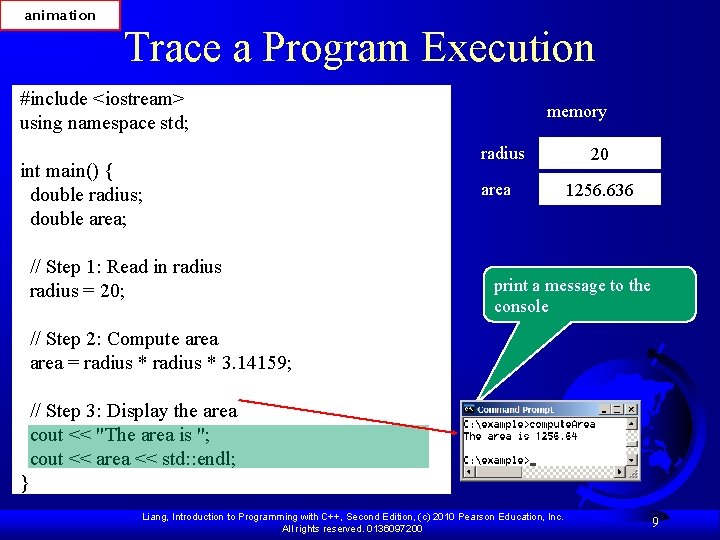
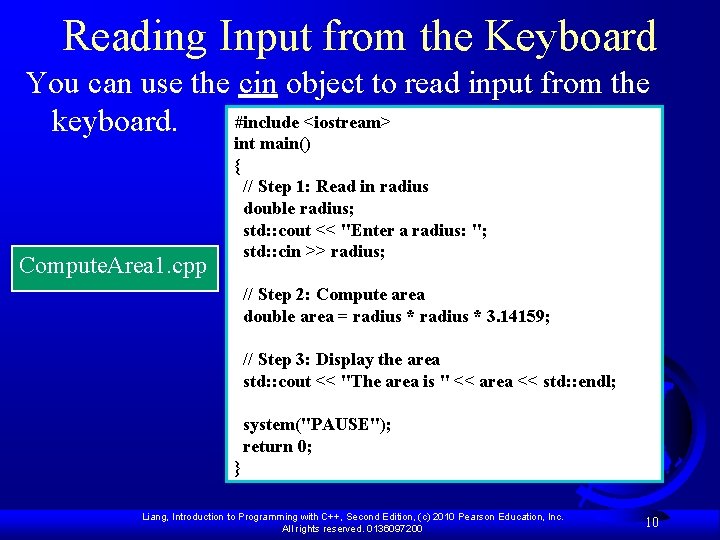
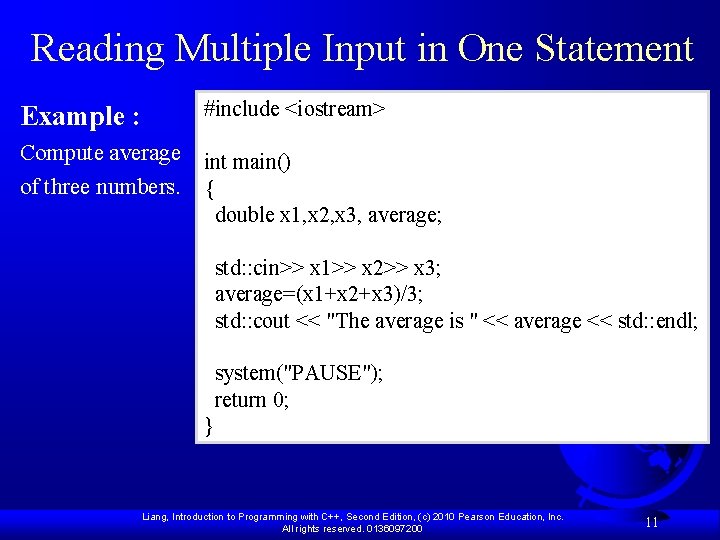
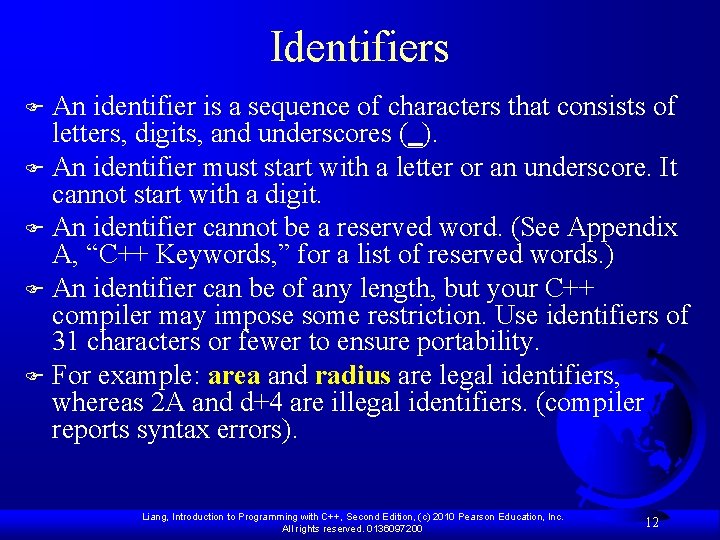
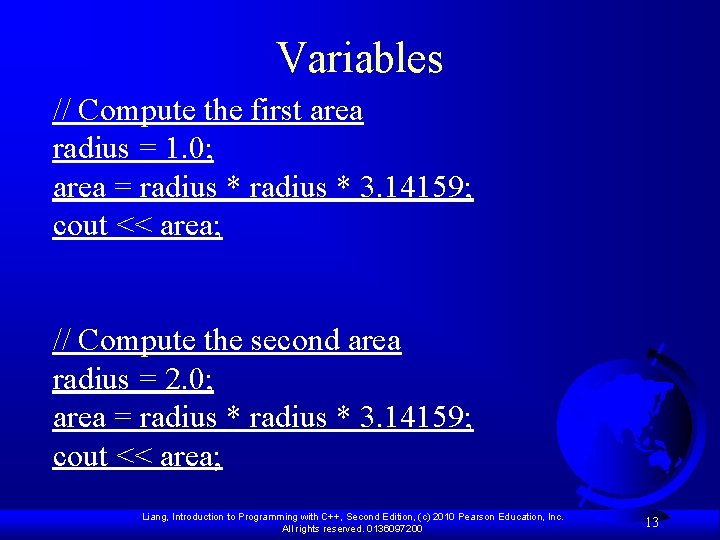
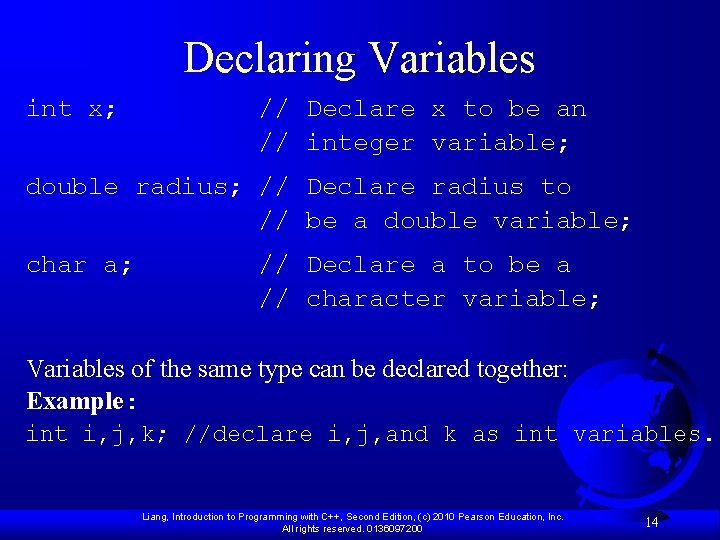
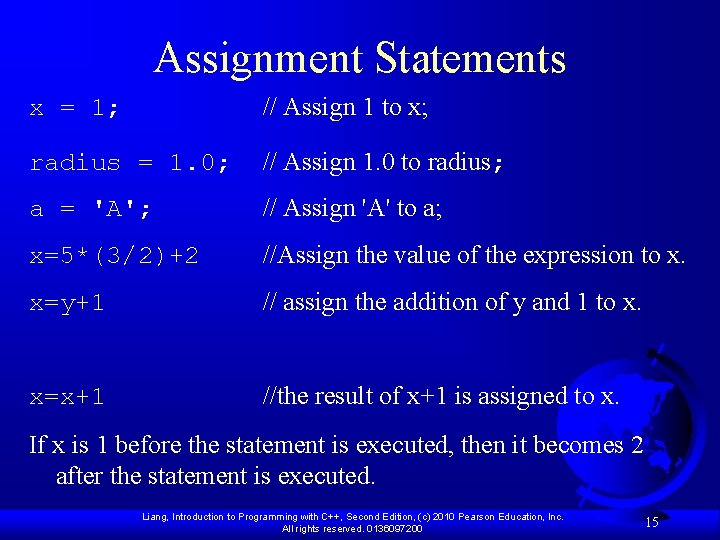
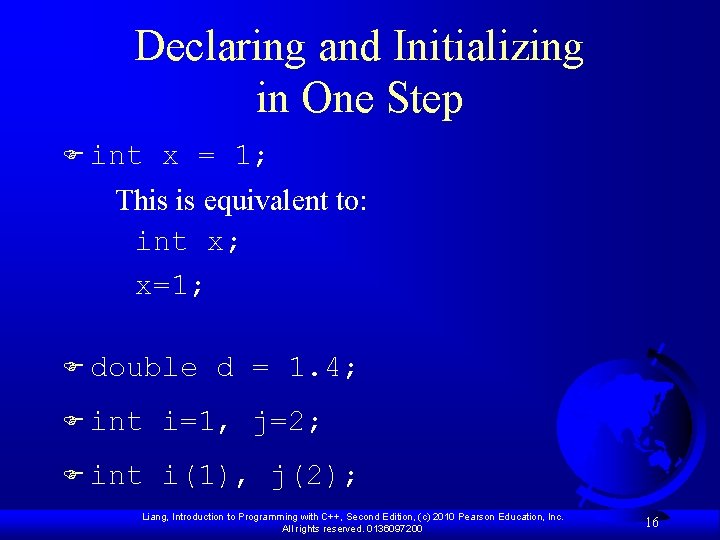
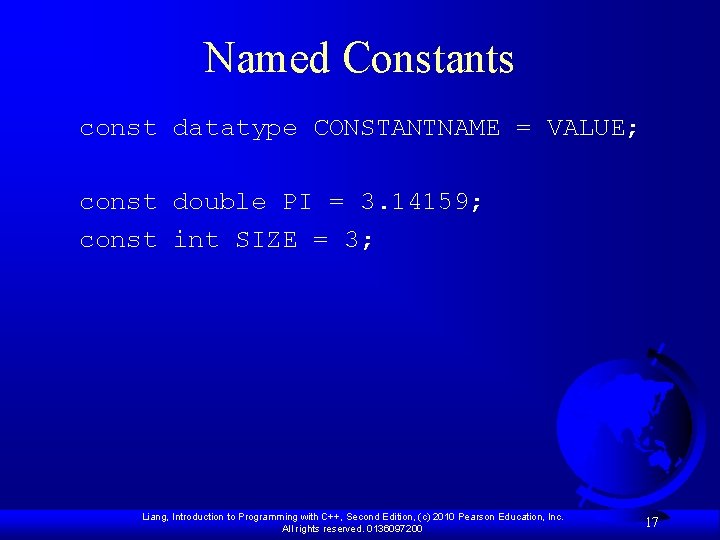
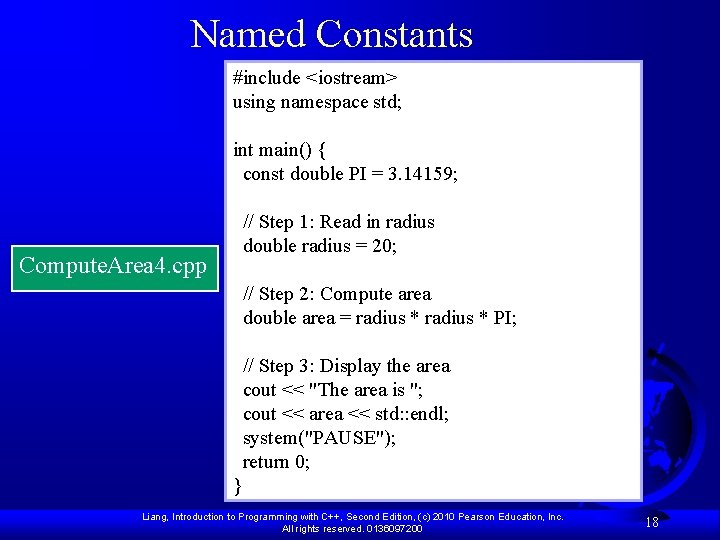
- Slides: 18
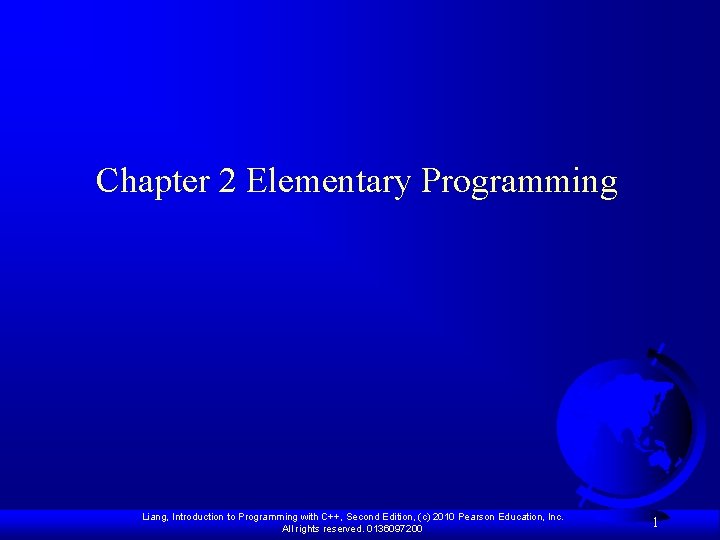
Chapter 2 Elementary Programming Liang, Introduction to Programming with C++, Second Edition, (c) 2010 Pearson Education, Inc. All rights reserved. 0136097200 1
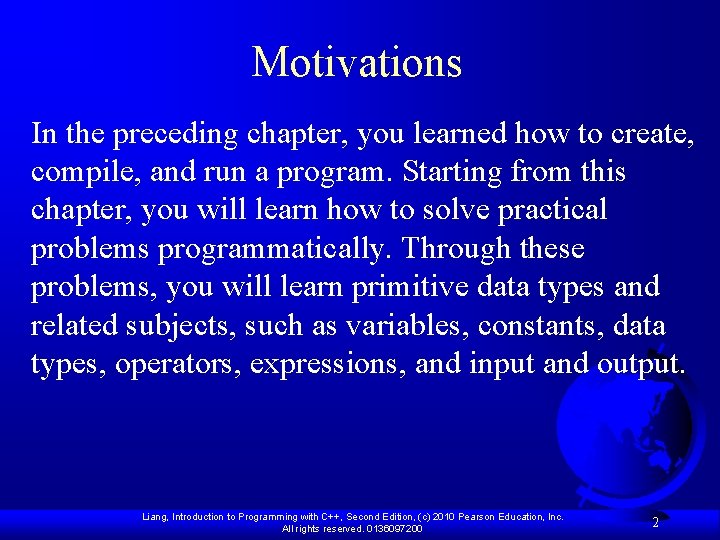
Motivations In the preceding chapter, you learned how to create, compile, and run a program. Starting from this chapter, you will learn how to solve practical problems programmatically. Through these problems, you will learn primitive data types and related subjects, such as variables, constants, data types, operators, expressions, and input and output. Liang, Introduction to Programming with C++, Second Edition, (c) 2010 Pearson Education, Inc. All rights reserved. 0136097200 2
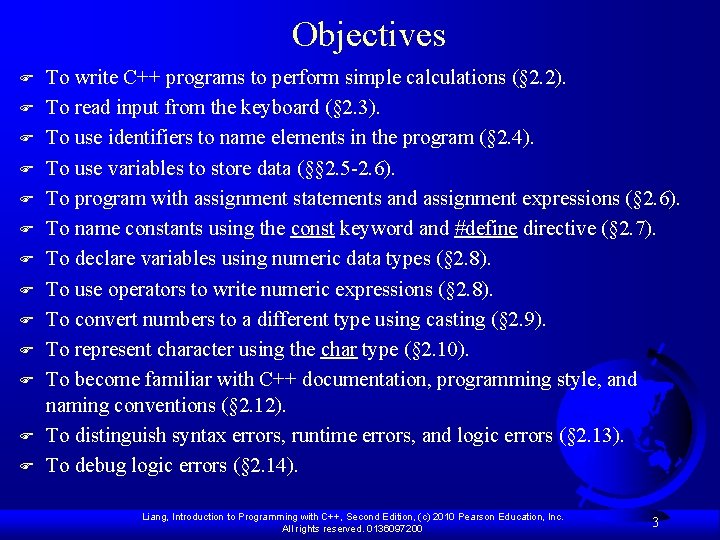
Objectives F F F F To write C++ programs to perform simple calculations (§ 2. 2). To read input from the keyboard (§ 2. 3). To use identifiers to name elements in the program (§ 2. 4). To use variables to store data (§§ 2. 5 -2. 6). To program with assignment statements and assignment expressions (§ 2. 6). To name constants using the const keyword and #define directive (§ 2. 7). To declare variables using numeric data types (§ 2. 8). To use operators to write numeric expressions (§ 2. 8). To convert numbers to a different type using casting (§ 2. 9). To represent character using the char type (§ 2. 10). To become familiar with C++ documentation, programming style, and naming conventions (§ 2. 12). To distinguish syntax errors, runtime errors, and logic errors (§ 2. 13). To debug logic errors (§ 2. 14). Liang, Introduction to Programming with C++, Second Edition, (c) 2010 Pearson Education, Inc. All rights reserved. 0136097200 3
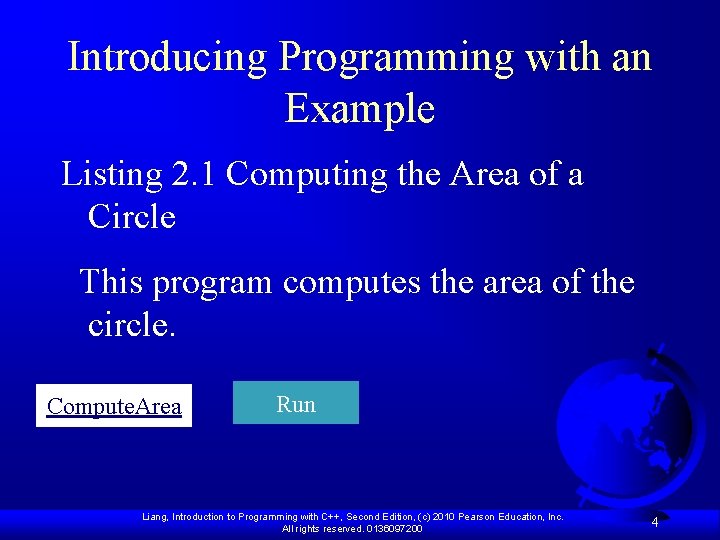
Introducing Programming with an Example Listing 2. 1 Computing the Area of a Circle This program computes the area of the circle. Compute. Area Run Liang, Introduction to Programming with C++, Second Edition, (c) 2010 Pearson Education, Inc. All rights reserved. 0136097200 4
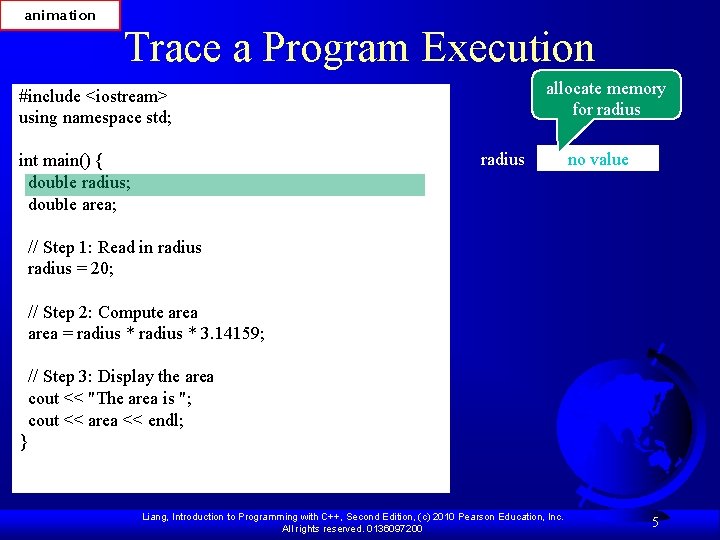
animation Trace a Program Execution allocate memory for radius #include <iostream> using namespace std; radius int main() { double radius; double area; no value // Step 1: Read in radius = 20; // Step 2: Compute area = radius * 3. 14159; // Step 3: Display the area cout << "The area is "; cout << area << endl; } Liang, Introduction to Programming with C++, Second Edition, (c) 2010 Pearson Education, Inc. All rights reserved. 0136097200 5
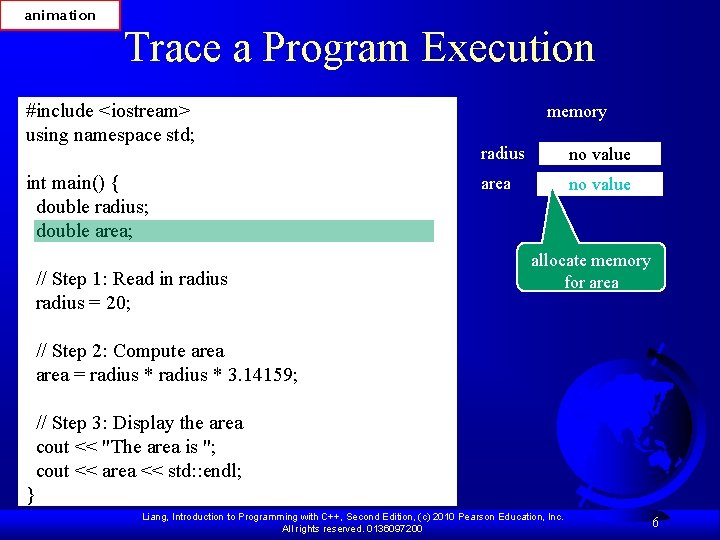
animation Trace a Program Execution #include <iostream> using namespace std; int main() { double radius; double area; // Step 1: Read in radius = 20; memory radius no value area no value allocate memory for area // Step 2: Compute area = radius * 3. 14159; // Step 3: Display the area cout << "The area is "; cout << area << std: : endl; } Liang, Introduction to Programming with C++, Second Edition, (c) 2010 Pearson Education, Inc. All rights reserved. 0136097200 6
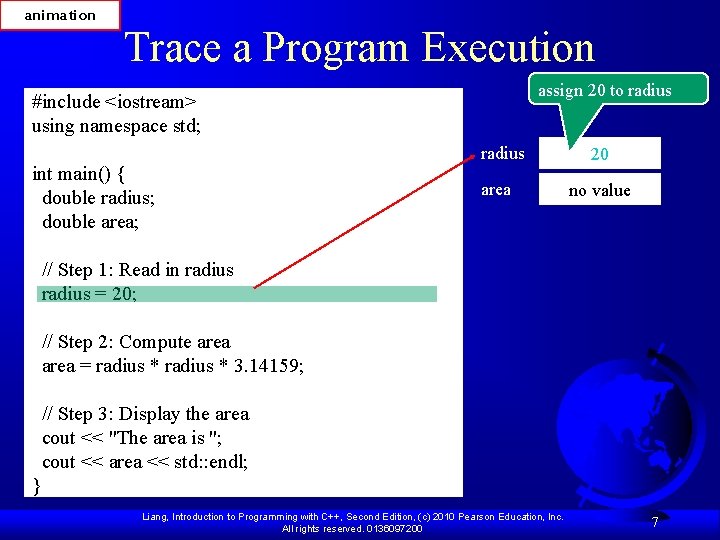
animation Trace a Program Execution assign 20 to radius #include <iostream> using namespace std; int main() { double radius; double area; radius area 20 no value // Step 1: Read in radius = 20; // Step 2: Compute area = radius * 3. 14159; // Step 3: Display the area cout << "The area is "; cout << area << std: : endl; } Liang, Introduction to Programming with C++, Second Edition, (c) 2010 Pearson Education, Inc. All rights reserved. 0136097200 7
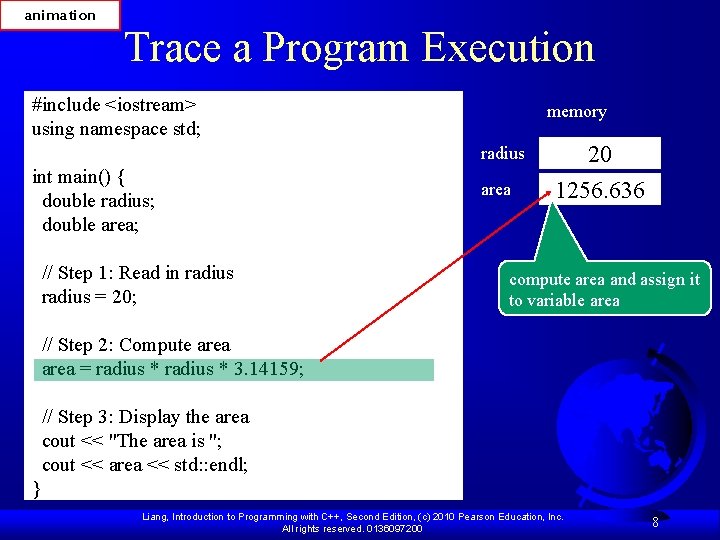
animation Trace a Program Execution #include <iostream> using namespace std; memory radius int main() { double radius; double area; // Step 1: Read in radius = 20; area 20 1256. 636 compute area and assign it to variable area // Step 2: Compute area = radius * 3. 14159; // Step 3: Display the area cout << "The area is "; cout << area << std: : endl; } Liang, Introduction to Programming with C++, Second Edition, (c) 2010 Pearson Education, Inc. All rights reserved. 0136097200 8
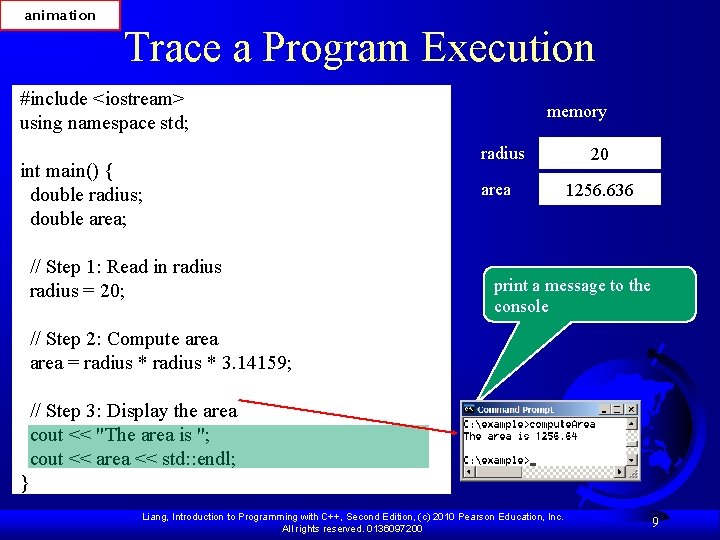
animation Trace a Program Execution #include <iostream> using namespace std; int main() { double radius; double area; // Step 1: Read in radius = 20; memory radius area 20 1256. 636 print a message to the console // Step 2: Compute area = radius * 3. 14159; // Step 3: Display the area cout << "The area is "; cout << area << std: : endl; } Liang, Introduction to Programming with C++, Second Edition, (c) 2010 Pearson Education, Inc. All rights reserved. 0136097200 9
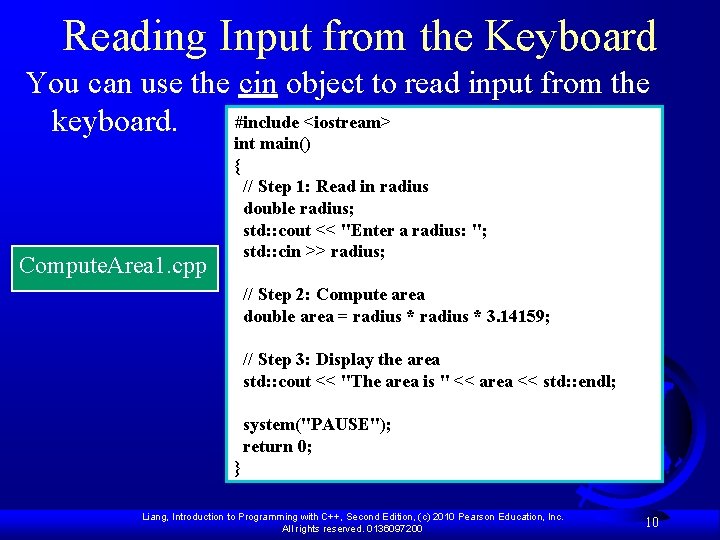
Reading Input from the Keyboard You can use the cin object to read input from the #include <iostream> keyboard. Compute. Area 1. cpp int main() { // Step 1: Read in radius double radius; std: : cout << "Enter a radius: "; std: : cin >> radius; // Step 2: Compute area double area = radius * 3. 14159; // Step 3: Display the area std: : cout << "The area is " << area << std: : endl; system("PAUSE"); return 0; } Liang, Introduction to Programming with C++, Second Edition, (c) 2010 Pearson Education, Inc. All rights reserved. 0136097200 10
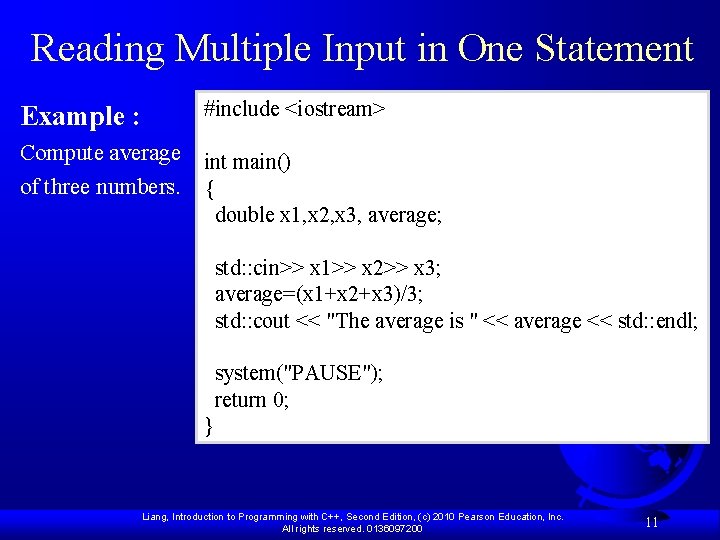
Reading Multiple Input in One Statement Example : #include <iostream> Compute average int main() of three numbers. { double x 1, x 2, x 3, average; std: : cin>> x 1>> x 2>> x 3; average=(x 1+x 2+x 3)/3; std: : cout << "The average is " << average << std: : endl; system("PAUSE"); return 0; } Liang, Introduction to Programming with C++, Second Edition, (c) 2010 Pearson Education, Inc. All rights reserved. 0136097200 11
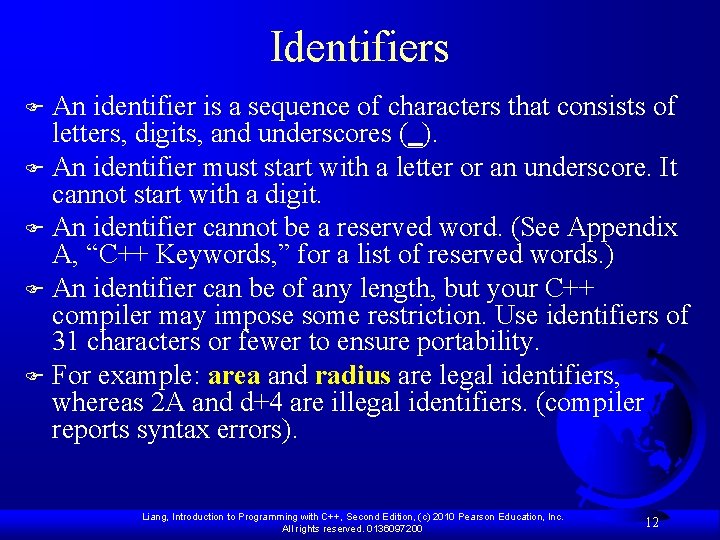
Identifiers An identifier is a sequence of characters that consists of letters, digits, and underscores (_). F An identifier must start with a letter or an underscore. It cannot start with a digit. F An identifier cannot be a reserved word. (See Appendix A, “C++ Keywords, ” for a list of reserved words. ) F An identifier can be of any length, but your C++ compiler may impose some restriction. Use identifiers of 31 characters or fewer to ensure portability. F For example: area and radius are legal identifiers, whereas 2 A and d+4 are illegal identifiers. (compiler reports syntax errors). F Liang, Introduction to Programming with C++, Second Edition, (c) 2010 Pearson Education, Inc. All rights reserved. 0136097200 12
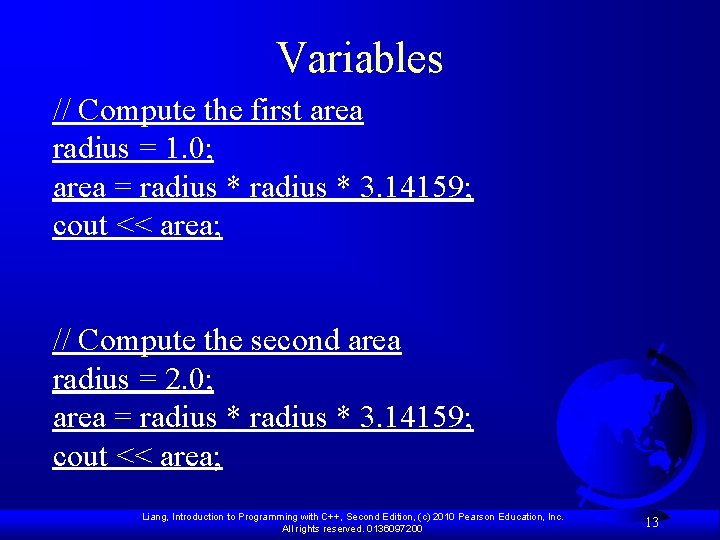
Variables // Compute the first area radius = 1. 0; area = radius * 3. 14159; cout << area; // Compute the second area radius = 2. 0; area = radius * 3. 14159; cout << area; Liang, Introduction to Programming with C++, Second Edition, (c) 2010 Pearson Education, Inc. All rights reserved. 0136097200 13
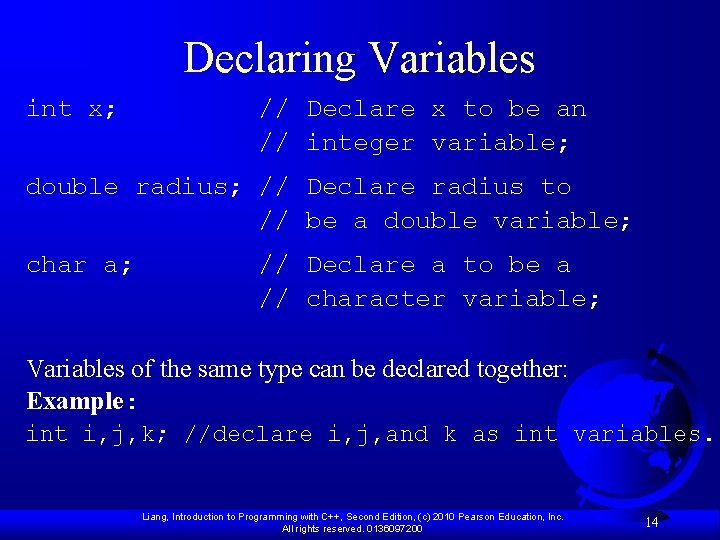
Declaring Variables int x; // Declare x to be an // integer variable; double radius; // Declare radius to // be a double variable; char a; // Declare a to be a // character variable; Variables of the same type can be declared together: Example: int i, j, k; //declare i, j, and k as int variables. Liang, Introduction to Programming with C++, Second Edition, (c) 2010 Pearson Education, Inc. All rights reserved. 0136097200 14
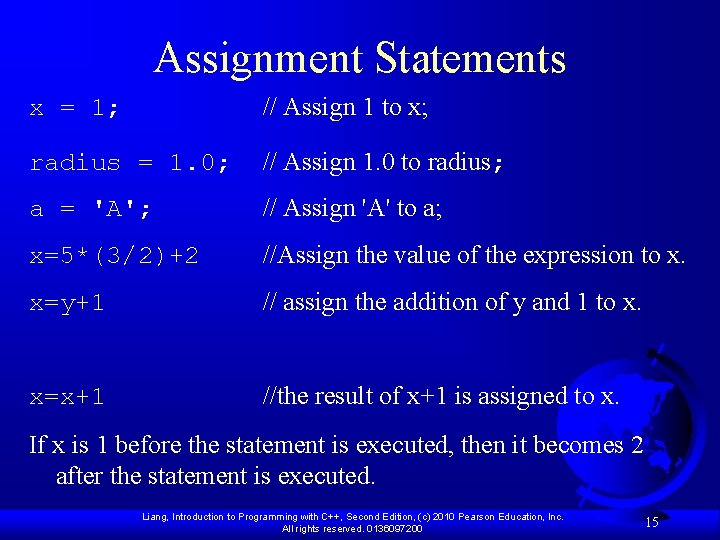
Assignment Statements x = 1; // Assign 1 to x; radius = 1. 0; // Assign 1. 0 to radius; a = 'A'; // Assign 'A' to a; x=5*(3/2)+2 //Assign the value of the expression to x. x=y+1 // assign the addition of y and 1 to x. x=x+1 //the result of x+1 is assigned to x. If x is 1 before the statement is executed, then it becomes 2 after the statement is executed. Liang, Introduction to Programming with C++, Second Edition, (c) 2010 Pearson Education, Inc. All rights reserved. 0136097200 15
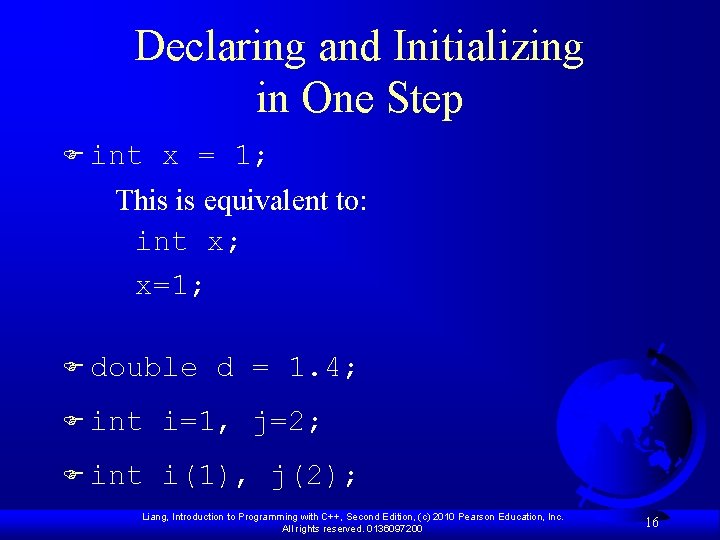
Declaring and Initializing in One Step F int x = 1; This is equivalent to: int x; x=1; F double d = 1. 4; F int i=1, j=2; F int i(1), j(2); Liang, Introduction to Programming with C++, Second Edition, (c) 2010 Pearson Education, Inc. All rights reserved. 0136097200 16
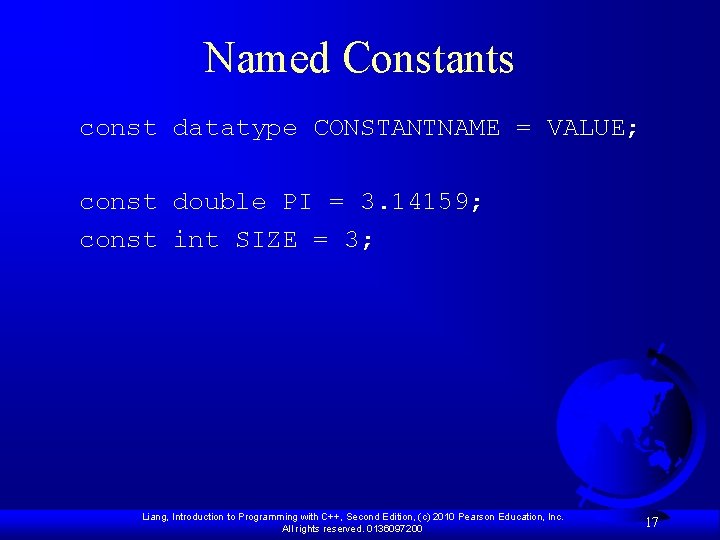
Named Constants const datatype CONSTANTNAME = VALUE; const double PI = 3. 14159; const int SIZE = 3; Liang, Introduction to Programming with C++, Second Edition, (c) 2010 Pearson Education, Inc. All rights reserved. 0136097200 17
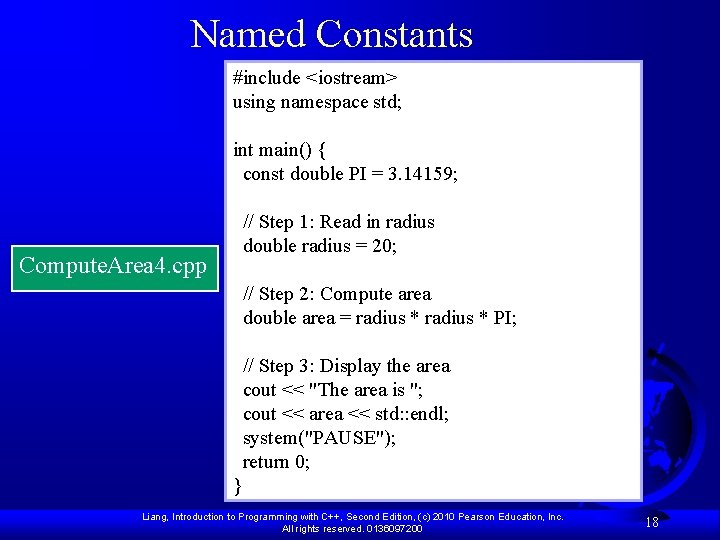
Named Constants #include <iostream> using namespace std; int main() { const double PI = 3. 14159; // Step 1: Read in radius double radius = 20; Compute. Area 4. cpp // Step 2: Compute area double area = radius * PI; // Step 3: Display the area cout << "The area is "; cout << area << std: : endl; system("PAUSE"); return 0; } Liang, Introduction to Programming with C++, Second Edition, (c) 2010 Pearson Education, Inc. All rights reserved. 0136097200 18
Elementary programming in java
Chapter 2 elementary programming
Richard seow yung liang
Zhuo shi wo li liang
Zhong han liang
Yuanxing liang
Amber liang
Liang fontdemo.java
Liang fontdemo.java
Cara mengindeks surat dalam sistem abjad
Zachary hensley
David teo choon liang
How was ma liang like?
Zong-liang yang
Liang jianhui
Zhang liang game
Chia liang cheng
Materi otk keuangan kelas 11 semester 2
Mechanical engineering umbc