How to Convert String to Integer in Python
- Method 1: Using the int() Function
- Method 2: Using the float() Function
- Method 3: Using Exception Handling
- Conclusion
- FAQ
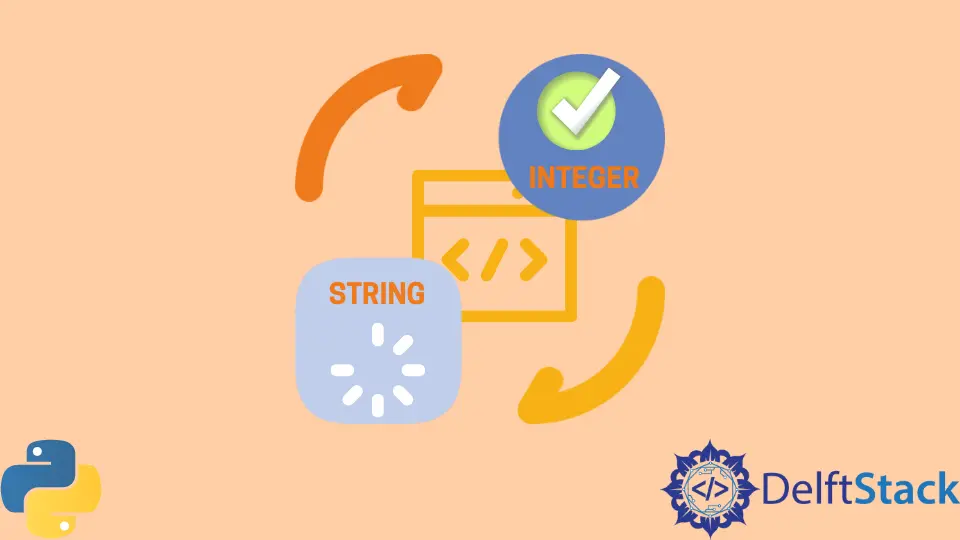
Converting strings to integers in Python is a fundamental skill that every programmer should master. Whether you’re processing user input, reading from files, or working with data from APIs, the ability to transform a string representation of a number into an integer is crucial.
In this tutorial, we will explore various methods to achieve this conversion efficiently and effectively. We’ll provide clear examples and explanations to ensure you understand each technique. By the end of this article, you will have a solid grasp of how to convert strings to integers in Python, enhancing your programming toolkit and enabling you to handle numerical data with confidence.
Method 1: Using the int() Function
One of the simplest and most commonly used methods to convert a string to an integer in Python is by utilizing the built-in int()
function. This function takes a string as an argument and returns its integer equivalent. It can handle both decimal and hexadecimal strings, making it versatile for various applications.
Here’s how it works:
pythonCopynumber_str = "123"
number_int = int(number_str)
Output:
textCopy123
The int()
function successfully converts the string “123” into the integer 123. If the string contains non-numeric characters, a ValueError
will be raised. For example, trying to convert “123abc” will result in an error. This method is straightforward and efficient, making it the go-to choice for many programmers when they need to convert strings to integers.
The int()
function can also take an optional second argument that specifies the base of the number system to be used. For instance, you can convert a binary string to an integer by passing 2 as the second argument:
pythonCopybinary_str = "1010"
binary_int = int(binary_str, 2)
Output:
textCopy10
In this case, the binary string “1010” is converted to the integer 10. This feature makes the int()
function particularly useful for handling different numeral systems.
Method 2: Using the float() Function
Another method to convert a string to an integer is by first converting it to a float using the float()
function and then casting it to an integer. While this method may seem a bit indirect, it can be useful in scenarios where you expect the string to represent a floating-point number.
Here’s how you can do it:
pythonCopynumber_str = "123.45"
number_float = float(number_str)
number_int = int(number_float)
Output:
textCopy123
In this example, the string “123.45” is first converted to a float, resulting in 123.45. When we cast this float to an integer, it truncates the decimal part and gives us 123. This method is particularly handy when dealing with strings that may contain decimal points, as it allows you to handle both integers and floats in a single conversion process.
However, it’s important to note that this method will always truncate the decimal portion, which may not be desirable in all situations. If you need to round the number instead of truncating it, you can use the round()
function before converting to an integer:
pythonCopynumber_str = "123.75"
number_float = float(number_str)
number_int = int(round(number_float))
Output:
textCopy124
By using round()
, the float 123.75 is rounded to 124 before the conversion. This method provides flexibility in handling string representations of both integers and floating-point numbers.
Method 3: Using Exception Handling
When converting strings to integers, it’s essential to be prepared for potential errors, especially when dealing with user input or external data sources. Using exception handling with a try
and except
block can help you manage these errors gracefully.
Here’s an example of how to implement this:
pythonCopynumber_str = "abc123"
try:
number_int = int(number_str)
except ValueError:
number_int = None
Output:
textCopyNone
In this case, we attempt to convert the string “abc123” to an integer. Since the string contains non-numeric characters, the int()
function raises a ValueError
. Instead of crashing the program, we catch this error in the except
block and set number_int
to None
.
This method is particularly useful when you expect that the input data may not always be valid. By using exception handling, you can provide feedback to the user or handle the error in a way that maintains the program’s stability. For instance, you could prompt the user to enter a valid number again or log the error for further analysis.
Exception handling is a best practice in programming, especially when dealing with external inputs, as it ensures that your application can handle unexpected situations without crashing.
Conclusion
Converting strings to integers in Python is a vital skill that every developer should possess. In this tutorial, we explored three effective methods: using the int()
function, converting to float first, and utilizing exception handling for error management. Each method has its advantages and can be applied based on the specific requirements of your project. By mastering these techniques, you’ll be better equipped to handle numerical data in your Python applications, ensuring robustness and reliability in your code.
FAQ
-
What happens if I try to convert a non-numeric string using int()?
If you try to convert a non-numeric string using the int() function, it will raise a ValueError. -
Can I convert a string with decimal points directly to an integer?
No, you cannot convert a string with decimal points directly to an integer. You must first convert it to a float and then cast it to an integer. -
Is there a way to handle errors when converting strings to integers?
Yes, you can use a try-except block to handle errors gracefully when converting strings to integers. -
Can the int() function handle different numeral systems?
Yes, the int() function can handle different numeral systems by specifying the base as the second argument. -
What is the difference between truncating and rounding when converting to an integer?
Truncating removes the decimal part without rounding, while rounding adjusts the number to the nearest integer.
Related Article - Python String
- How to Remove Commas From String in Python
- How to Check a String Is Empty in a Pythonic Way
- How to Convert a String to Variable Name in Python
- How to Remove Whitespace From a String in Python
- How to Extract Numbers From a String in Python
- How to Convert String to Datetime in Python