How to Convert CSV File to JSON File in Python
- Why Convert CSV to JSON?
- Method 1: Using the pandas Library
- Method 2: Using the csv and json Libraries
- Method 3: Using the json_normalize Function
- Conclusion
- FAQ
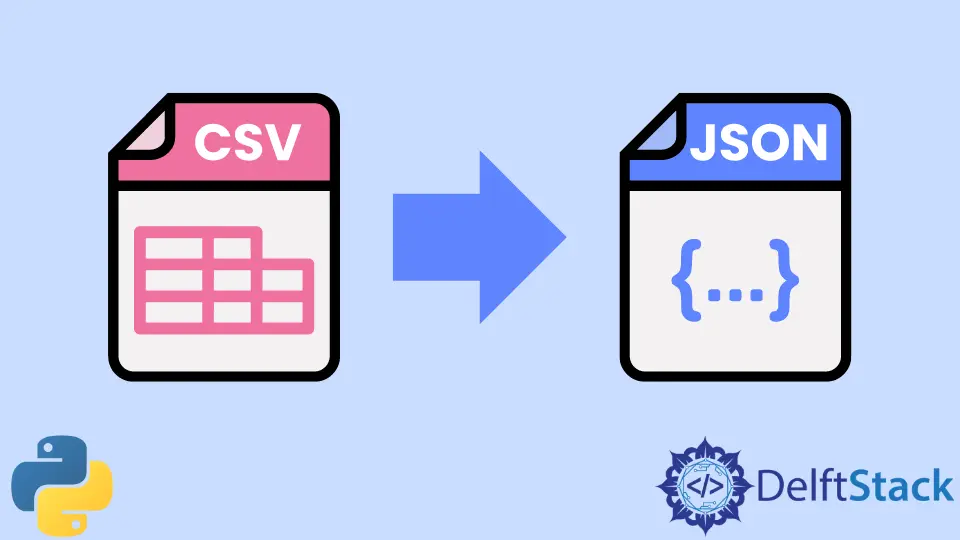
Converting a CSV file to a JSON file in Python is a common task that many developers encounter. Whether you’re working with data analysis, web development, or data migration, knowing how to perform this conversion can save you time and effort.
In this tutorial, we will walk through the process step-by-step, providing clear examples and explanations along the way. By the end, you’ll have a solid understanding of how to easily convert CSV data into JSON format using Python. This knowledge will not only enhance your programming skills but also improve your ability to handle data in various applications.
Why Convert CSV to JSON?
CSV (Comma-Separated Values) is a popular format for storing tabular data. It’s simple and easy to read. However, when it comes to web applications or APIs, JSON (JavaScript Object Notation) is often preferred due to its lightweight structure and ease of use with JavaScript. Converting CSV to JSON allows you to leverage the strengths of both formats, making your data more versatile and easier to manipulate in applications.
Method 1: Using the pandas Library
One of the most efficient ways to convert a CSV file to JSON in Python is by using the pandas library. Pandas is a powerful data manipulation and analysis library that provides a simple interface for handling data. Here’s how you can do it:
pythonCopyimport pandas as pd
# Load the CSV file
csv_file = 'data.csv'
data = pd.read_csv(csv_file)
# Convert to JSON format
json_file = 'data.json'
data.to_json(json_file, orient='records', lines=True)
In this code, we start by importing the pandas library. We then load the CSV file into a DataFrame using pd.read_csv()
. After that, we convert the DataFrame to JSON format using the to_json()
method, specifying the file name and the desired orientation. The orient='records'
option creates a list of records, while lines=True
ensures that each record is on a new line in the JSON file.
Output:
textCopy[{"column1":"value1","column2":"value2"}, {"column1":"value3","column2":"value4"}]
Using pandas not only simplifies the conversion process but also allows you to handle larger datasets efficiently. The library is optimized for performance, making it an excellent choice for data manipulation tasks.
Method 2: Using the csv and json Libraries
If you prefer not to use external libraries, you can achieve the same result using Python’s built-in csv
and json
libraries. This method gives you more control over the conversion process and can be helpful for smaller datasets or specific formatting needs.
pythonCopyimport csv
import json
# Load the CSV file
csv_file = 'data.csv'
json_file = 'data.json'
with open(csv_file, mode='r') as file:
csv_reader = csv.DictReader(file)
data = [row for row in csv_reader]
# Write to JSON file
with open(json_file, mode='w') as file:
json.dump(data, file, indent=4)
In this example, we first import the necessary libraries. We open the CSV file and use csv.DictReader()
to read the rows into a list of dictionaries, where each dictionary corresponds to a row in the CSV. Finally, we write the list to a JSON file using json.dump()
, specifying an indentation level for better readability.
Output:
textCopy[
{
"column1": "value1",
"column2": "value2"
},
{
"column1": "value3",
"column2": "value4"
}
]
This method is particularly useful when you want to customize how the data is read or written. It also allows you to handle specific edge cases, such as missing values or different data types. While it may require a bit more code than using pandas, it gives you complete control over the conversion process.
Method 3: Using the json_normalize Function
If your CSV data has a nested structure or if you want to flatten it into a more usable format, you can use the json_normalize
function from pandas. This function is great for converting complex data structures into a flat JSON format.
pythonCopyimport pandas as pd
# Load the CSV file
csv_file = 'data_nested.csv'
data = pd.read_csv(csv_file)
# Normalize and convert to JSON
json_file = 'data_flat.json'
normalized_data = pd.json_normalize(data)
normalized_data.to_json(json_file, orient='records', lines=True)
In this code snippet, we again use pandas to read the CSV file. After loading the data into a DataFrame, we apply the pd.json_normalize()
function to flatten the data. Finally, we convert the normalized data to JSON format and save it to a file.
Output:
textCopy[{"nested_column1":"value1","nested_column2":"value2"}, {"nested_column1":"value3","nested_column2":"value4"}]
This method is particularly useful when you’re dealing with hierarchical data or when you want to simplify complex data structures. The json_normalize
function takes care of flattening the data for you, making it easier to work with in JSON format.
Conclusion
Converting CSV files to JSON in Python is a straightforward task that can be accomplished using various methods. Whether you prefer the simplicity of pandas or the control provided by the built-in libraries, each approach has its advantages. Understanding these methods will not only enhance your data manipulation skills but also prepare you for handling real-world data scenarios. With this knowledge, you can efficiently convert data formats and make your applications more robust and versatile.
FAQ
-
What is the main difference between CSV and JSON?
CSV is a simple, tabular format, while JSON is a structured format that supports nested data and is more suitable for web applications. -
Do I need to install any libraries to convert CSV to JSON in Python?
You only need to install pandas if you choose to use it. The csv and json libraries are built-in and do not require installation. -
Can I convert large CSV files to JSON using Python?
Yes, Python can handle large CSV files, especially when using pandas, which is optimized for performance. -
What is the best method to convert CSV to JSON?
The best method depends on your specific needs. For simple conversions, pandas is often the easiest, while the built-in libraries offer more control. -
Can I customize the JSON output format?
Yes, you can customize the output format by adjusting the parameters in the pandas or json libraries during the conversion process.
Related Article - Python CSV
- How to Import Multiple CSV Files Into Pandas and Concatenate Into One DataFrame
- How to Split CSV Into Multiple Files in Python
- How to Compare Two CSV Files and Print Differences Using Python
- How to Convert XLSX to CSV File in Python
- How to Write List to CSV Columns in Python
- How to Write to CSV Line by Line in Python