C++ Nested if-else Statement
Nested if-else statements are those statements in which there is an if statement inside another if else. We use nested if-else statements when we want to implement multilayer conditions (condition inside the condition inside the condition and so on). C++ allows any number of nesting levels.
Let’s take a look at a simple example:
#include <iostream>
using namespace std;
int main() {
int n = 6;
// Outer if statement
if (n % 2 == 0) {
// Inner if statement
if (n % 3 == 0) {
cout << "Divisible by 2 and 3";
}
else {
cout << "Divisible by 2 but not 3";
}
}
else {
cout << "Not Divisible by 2";
}
return 0;
}
Output
Divisible by 2 and 3
Explanation: In the above program, the outer if statement checks whether n is divisible by 2 and the inner if statement checks if n is divisible by 3. But as inner if is nested, the divisibly check with 3 is only performed if n is divisible by 2.
Basic Syntax of Nested if-else
The nesting of if-else depends on the situation but a general syntax can be defined as:
if(condition1) {
if(condition2) {
// Statement 1
}
else {
// Statement 2
}
}
else {
if(condition3) {
// Statement 3
}
else {
// Statement 4
}
}
In the above syntax of nested if-else statements, the inner if-else statement is executed only if ‘condition1’ becomes true otherwise else statement will be executed and this same rule will be applicable for inner if-else statements.
Flowchart of Nested if-else
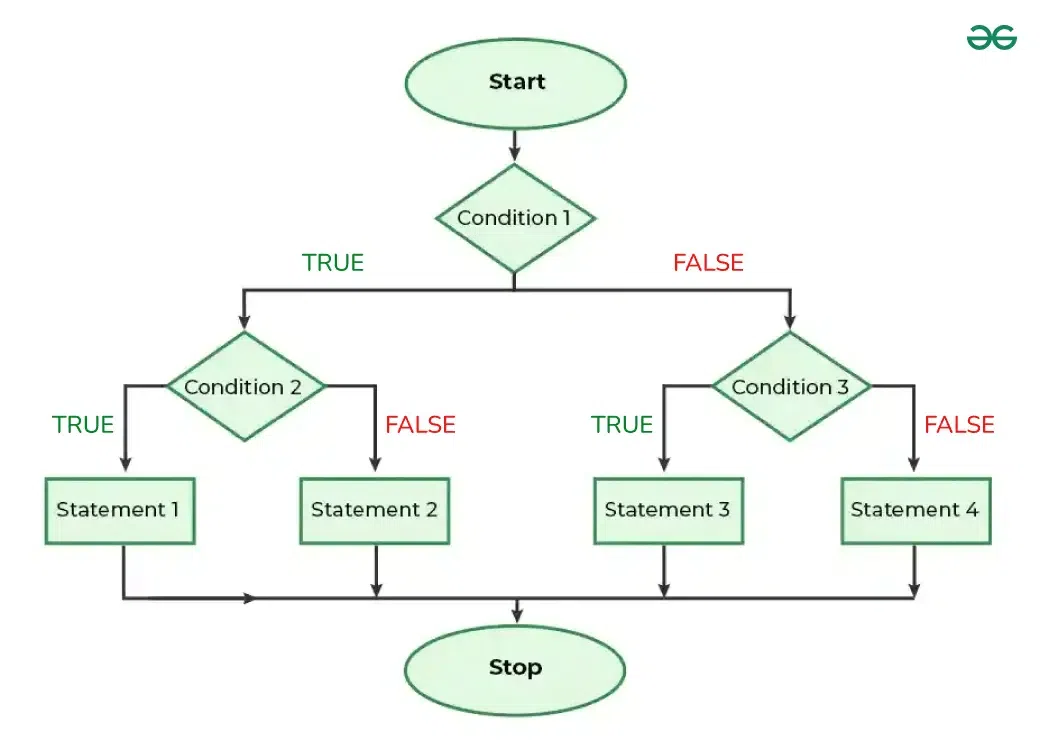
Examples of Nested if-else
The following C++ programs demonstrate the use of nested if-else statements.
Check the Greatest Among Three Numbers
#include <iostream>
using namespace std;
int main() {
int a = 10, b = 2, c = 6;
// Outermost if else
if (a < b) {
// Nested if else
if (c < b) {
cout << b;
}
else {
cout << c;
}
}
else {
// Nested if else
if (c < a) {
cout << a;
}
else {
cout << c;
}
}
return 0;
}
Output
10
Check if the Given Year is a Leap Year
A year is a leap year if it follows the condition, the year should be evenly divisible by 4 then the year should be evenly divisible by 100 then lastly year should evenly divisible by 400 otherwise the year is not a leap year.
#include <iostream>
using namespace std;
int main() {
int year = 2023;
// Outer if-else statement to check divisiblity with 0
if (year % 4 == 0) {
// First nested if-else statement to check
// divisiblity with 100
if (year % 100 == 0) {
// Second nested if-else statement to check
// divisiblity with 400
if (year % 400 == 0) {
cout << year << " is a leap year.";
}
else {
cout << year << " is not a leap year.";
}
}
else {
cout << year << " is a leap year.";
}
}
else {
cout << year << " is not a leap year.";
}
return 0;
}
Output
2023 is not a leap year.