Decision Making in C (if , if..else, Nested if, if-else-if )
Last Updated :
10 Jan, 2025
The conditional statements (also known as decision control structures) such as if, if else, switch, etc. are used for decision-making purposes in C programs.
They are also known as Decision-Making Statements and are used to evaluate one or more conditions and make the decision whether to execute a set of statements or not. These decision-making statements in programming languages decide the direction of the flow of program execution.
Need of Conditional Statements
There come situations in real life when we need to make some decisions and based on these decisions, we decide what should we do next. Similar situations arise in programming also where we need to make some decisions and based on these decisions we will execute the next block of code. For example, in C if x occurs then execute y else execute z. There can also be multiple conditions like in C if x occurs then execute p, else if condition y occurs execute q, else execute r. This condition of C else-if is one of the many ways of importing multiple conditions.
Types of Conditional Statements in C

Following are the decision-making statements available in C:
- if Statement
- if-else Statement
- Nested if Statement
- if-else-if Ladder
- switch Statement
- Conditional Operator
- Jump Statements:
Let’s discuss each of them one by one.
1. if in C
The if statement is the most simple decision-making statement. It is used to decide whether a certain statement or block of statements will be executed or not i.e if a certain condition is true then a block of statements is executed otherwise not.
Syntax of if Statement
if(condition)
{
// Statements to execute if
// condition is true
}
Here, the condition after evaluation will be either true or false. C if statement accepts boolean values – if the value is true then it will execute the block of statements below it otherwise not. If we do not provide the curly braces ‘{‘ and ‘}’ after if(condition) then by default if statement will consider the first immediately below statement to be inside its block.
Flowchart of if Statement

Flow Diagram of if Statement
Example of if in C
C
// C program to illustrate If statement
#include <stdio.h>
int main()
{
int i = 10;
if (i > 15) {
printf("10 is greater than 15");
}
printf("I am Not in if");
}
As the condition present in the if statement is false. So, the block below the if statement is not executed.
2. if-else in C
The if statement alone tells us that if a condition is true it will execute a block of statements and if the condition is false it won’t. But what if we want to do something else when the condition is false? Here comes the C else statement. We can use the else statement with the if statement to execute a block of code when the condition is false. The if-else statement consists of two blocks, one for false expression and one for true expression.
Syntax of if else in C
if (condition)
{
// Executes this block if
// condition is true
}
else
{
// Executes this block if
// condition is false
}
Flowchart of if-else Statement

Flow Diagram of if else
Example of if-else
C
// C program to illustrate If statement
#include <stdio.h>
int main()
{
int i = 20;
if (i < 15) {
printf("i is smaller than 15");
}
else {
printf("i is greater than 15");
}
return 0;
}
Outputi is greater than 15
The block of code following the else statement is executed as the condition present in the if statement is false.
3. Nested if-else in C
A nested if in C is an if statement that is the target of another if statement. Nested if statements mean an if statement inside another if statement. Yes, C allow us to nested if statements within if statements, i.e, we can place an if statement inside another if statement.
Syntax of Nested if-else
if (condition1)
{
// Executes when condition1 is true
if (condition_2)
{
// statement 1
}
else
{
// Statement 2
}
}
else {
if (condition_3)
{
// statement 3
}
else
{
// Statement 4
}
}
The below flowchart helps in visualize the above syntax.
Flowchart of Nested if-else
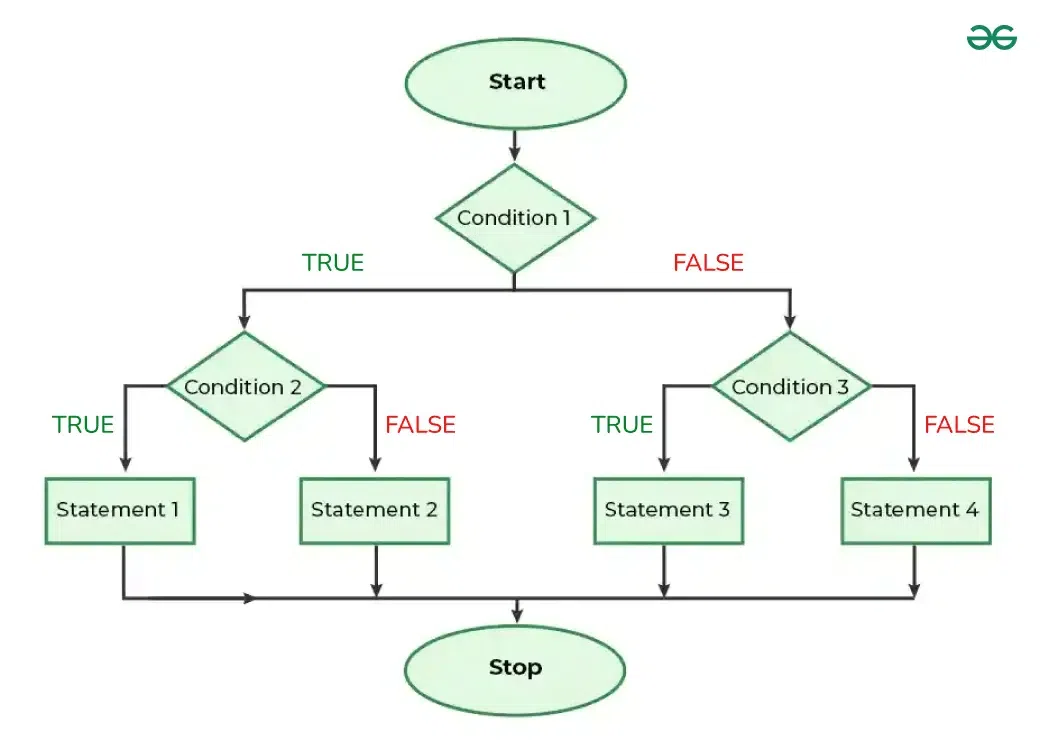
Example of Nested if-else
C
// C program to illustrate nested-if statement
#include <stdio.h>
int main()
{
int i = 10;
if (i == 10) {
// First if statement
if (i < 15)
printf("i is smaller than 15\n");
// Nested - if statement
// Will only be executed if statement above
// is true
if (i < 12)
printf("i is smaller than 12 too\n");
else
printf("i is greater than 15");
}
else {
if (i == 20) {
// Nested - if statement
// Will only be executed if statement above
// is true
if (i < 22)
printf("i is smaller than 22 too\n");
else
printf("i is greater than 25");
}
}
return 0;
}
Outputi is smaller than 15
i is smaller than 12 too
4. if-else-if Ladder in C
The if else if statements are used when the user has to decide among multiple options. The C if statements are executed from the top down. As soon as one of the conditions controlling the if is true, the statement associated with that if is executed, and the rest of the C else-if ladder is bypassed. If none of the conditions is true, then the final else statement will be executed. if-else-if ladder is similar to the switch statement.
Syntax of if-else-if Ladder
if (condition)
statement;
else if (condition)
statement;
.
.
else
statement;
Flowchart of if-else-if Ladder
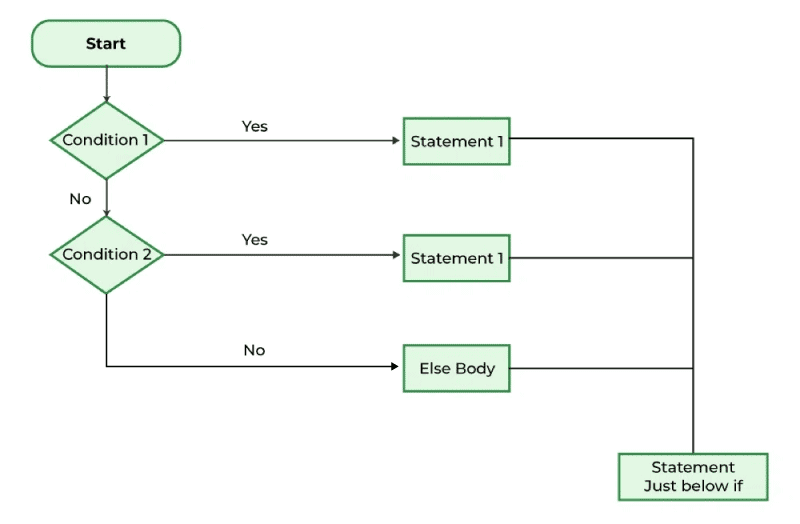
Flow Diagram of if-else-if
Example of if-else-if Ladder
C
// C program to illustrate nested-if statement
#include <stdio.h>
int main()
{
int i = 20;
if (i == 10)
printf("i is 10");
else if (i == 15)
printf("i is 15");
else if (i == 20)
printf("i is 20");
else
printf("i is not present");
}
5. switch Statement in C
The switch case statement is an alternative to the if else if ladder that can be used to execute the conditional code based on the value of the variable specified in the switch statement. The switch block consists of cases to be executed based on the value of the switch variable.
Syntax of switch
switch (expression) {
case value1:
statements;
case value2:
statements;
....
....
....
default:
statements;
}
Note: The switch expression should evaluate to either integer or character. It cannot evaluate any other data type.
Flowchart of switch

Flowchart of switch in C
Example of switch Statement
C
// C Program to illustrate the use of switch statement
#include <stdio.h>
int main()
{
// variable to be used in switch statement
int var = 2;
// declaring switch cases
switch (var) {
case 1:
printf("Case 1 is executed");
break;
case 2:
printf("Case 2 is executed");
break;
default:
printf("Default Case is executed");
break;
}
return 0;
}
6. Conditional Operator in C
The conditional operator is used to add conditional code in our program. It is similar to the if-else statement. It is also known as the ternary operator as it works on three operands.
Syntax of Conditional Operator
(condition) ? [true_statements] : [false_statements];
Flowchart of Conditional Operator

Flow Diagram of Conditional Operator
Example of Conditional Operator
C
// C Program to illustrate the use of conditional operator
#include <stdio.h>
// driver code
int main()
{
int var;
int flag = 0;
// using conditional operator to assign the value to var
// according to the value of flag
var = flag == 0 ? 25 : -25;
printf("Value of var when flag is 0: %d\n", var);
// changing the value of flag
flag = 1;
// again assigning the value to var using same statement
var = flag == 0 ? 25 : -25;
printf("Value of var when flag is NOT 0: %d", var);
return 0;
}
OutputValue of var when flag is 0: 25
Value of var when flag is NOT 0: -25
7. Jump Statements in C
These statements are used in C for the unconditional flow of control throughout the functions in a program. They support four types of jump statements:
A) break
This loop control statement is used to terminate the loop. As soon as the break statement is encountered from within a loop, the loop iterations stop there, and control returns from the loop immediately to the first statement after the loop.
Syntax of break
break;
Basically, break statements are used in situations when we are not sure about the actual number of iterations for the loop or we want to terminate the loop based on some condition.
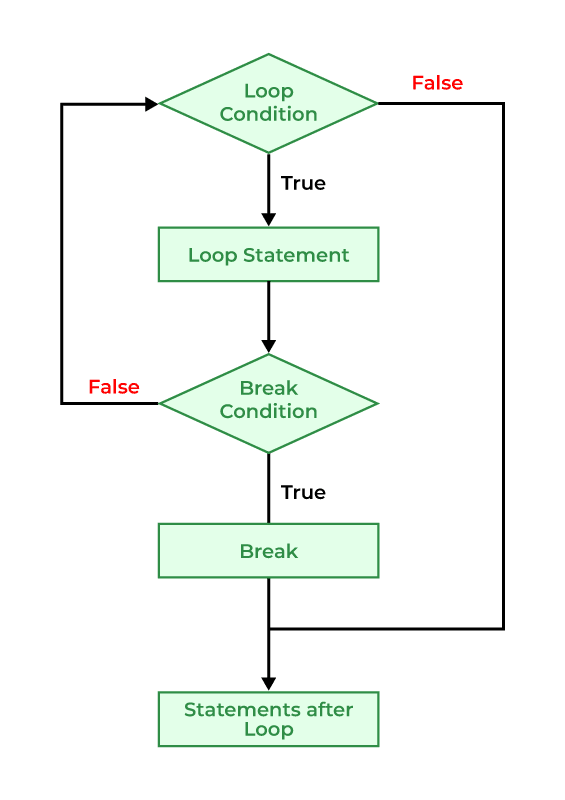
Example of break
C
// C program to illustrate
// to show usage of break
// statement
#include <stdio.h>
void findElement(int arr[], int size, int key)
{
// loop to traverse array and search for key
for (int i = 0; i < size; i++) {
if (arr[i] == key) {
printf("Element found at position: %d",
(i + 1));
break;
}
}
}
int main()
{
int arr[] = { 1, 2, 3, 4, 5, 6 };
// no of elements
int n = 6;
// key to be searched
int key = 3;
// Calling function to find the key
findElement(arr, n, key);
return 0;
}
OutputElement found at position: 3
B) continue
This loop control statement is just like the break statement. The continue statement is opposite to that of the break statement, instead of terminating the loop, it forces to execute the next iteration of the loop.
As the name suggests the continue statement forces the loop to continue or execute the next iteration. When the continue statement is executed in the loop, the code inside the loop following the continue statement will be skipped and the next iteration of the loop will begin.
Syntax of continue
continue;
Flowchart of Continue

Flow Diagram of C continue Statement
Example of continue
C
// C program to explain the use
// of continue statement
#include <stdio.h>
int main()
{
// loop from 1 to 10
for (int i = 1; i <= 10; i++) {
// If i is equals to 6,
// continue to next iteration
// without printing
if (i == 6)
continue;
else
// otherwise print the value of i
printf("%d ", i);
}
return 0;
}
If you create a variable in if-else in C, it will be local to that if/else block only. You can use global variables inside the if/else block. If the name of the variable you created in if/else is as same as any global variable then priority will be given to the `local variable`.
C
#include <stdio.h>
int main()
{
int gfg = 0; // local variable for main
printf("Before if-else block %d\n", gfg);
if (1) {
int gfg = 100; // new local variable of if block
printf("if block %d\n", gfg);
}
printf("After if block %d", gfg);
return 0;
}
OutputBefore if-else block 0
if block 100
After if block 0
C) goto
The goto statement in C also referred to as the unconditional jump statement can be used to jump from one point to another within a function.
Syntax of goto
Syntax1 | Syntax2
----------------------------
goto label; | label:
. | .
. | .
. | .
label: | goto label;
In the above syntax, the first line tells the compiler to go to or jump to the statement marked as a label. Here, a label is a user-defined identifier that indicates the target statement. The statement immediately followed after ‘label:’ is the destination statement. The ‘label:’ can also appear before the ‘goto label;’ statement in the above syntax.
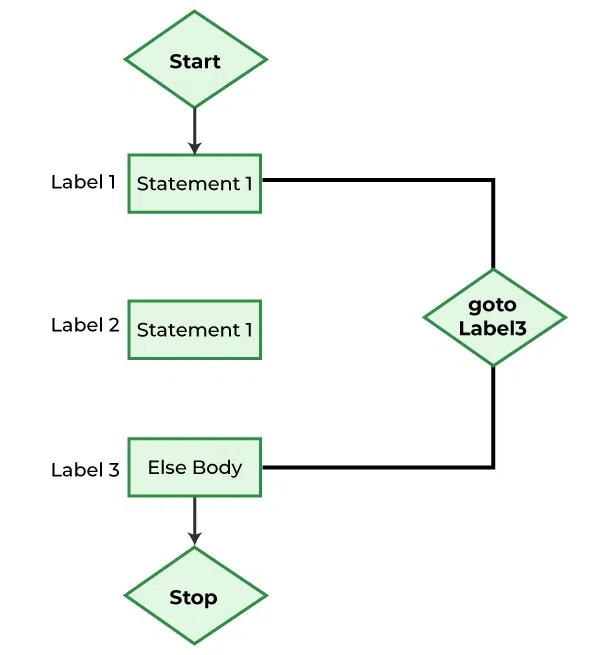
Flowchart of goto Statement
Examples of goto
C
// C program to print numbers
// from 1 to 10 using goto
// statement
#include <stdio.h>
// function to print numbers from 1 to 10
void printNumbers()
{
int n = 1;
label:
printf("%d ", n);
n++;
if (n <= 10)
goto label;
}
// Driver program to test above function
int main()
{
printNumbers();
return 0;
}
Output1 2 3 4 5 6 7 8 9 10
D) return
The return in C returns the flow of the execution to the function from where it is called. This statement does not mandatorily need any conditional statements. As soon as the statement is executed, the flow of the program stops immediately and returns the control from where it was called. The return statement may or may not return anything for a void function, but for a non-void function, a return value must be returned.
Flowchart of return

Flow Diagram of return
Syntax of return
return [expression];
Example of return
C
// C code to illustrate return
// statement
#include <stdio.h>
// non-void return type
// function to calculate sum
int SUM(int a, int b)
{
int s1 = a + b;
return s1;
}
// returns void
// function to print
void Print(int s2)
{
printf("The sum is %d", s2);
return;
}
int main()
{
int num1 = 10;
int num2 = 10;
int sum_of = SUM(num1, num2);
Print(sum_of);
return 0;
}
Similar Reads
C Programming Language Tutorial
C is a general-purpose, procedural, and middle-level programming language used for developing computer software, system programming, applications, games, and more. Known for its simplicity and efficiency, C is an excellent choice for beginners as it provides a strong foundation in programming concep
6 min read
C Basics
C Language Introduction
C is a procedural programming language initially developed by Dennis Ritchie in the year 1972 at Bell Laboratories of AT&T Labs. It was mainly developed as a system programming language to write the UNIX operating system. The main features of the C language include: General Purpose and PortableL
6 min read
Features of C Programming Language
C is a procedural programming language. It was initially developed by Dennis Ritchie in the year 1972. It was mainly developed as a system programming language to write an operating system. The main features of C language include low-level access to memory, a simple set of keywords, and a clean styl
3 min read
C Programming Language Standard
Introduction:The C programming language has several standard versions, with the most commonly used ones being C89/C90, C99, C11, and C18. C89/C90 (ANSI C or ISO C) was the first standardized version of the language, released in 1989 and 1990, respectively. This standard introduced many of the featur
6 min read
C Hello World Program
The âHello Worldâ program is the first step towards learning any programming language. It is also one of the simplest programs that is used to introduce aspiring programmers to the programming language. It typically outputs the text "Hello, World!" to the console screen. C Program to Print "Hello Wo
1 min read
Compiling a C Program: Behind the Scenes
The compilation is the process of converting the source code of the C language into machine code. As C is a mid-level language, it needs a compiler to convert it into an executable code so that the program can be run on our machine. The C program goes through the following phases during compilation:
4 min read
C Comments
The comments in C are human-readable explanations or notes in the source code of a C program. A comment makes the program easier to read and understand. These are the statements that are not executed by the compiler or an interpreter. Example: [GFGTABS] C #include <stdio.h> int main() { // Thi
3 min read
Tokens in C
In C programming, tokens are the smallest units in a program that have meaningful representations. Tokens are the building blocks of a C program, and they are recognized by the C compiler to form valid expressions and statements. Tokens can be classified into various categories, each with specific r
4 min read
Keywords in C
In C Programming language, there are many rules so to avoid different types of errors. One of such rule is not able to declare variable names with auto, long, etc. This is all because these are keywords. Let us check all keywords in C language. What are Keywords?Keywords are predefined or reserved w
13 min read
C Variables and Constants
C Variables
In C, variable is a name given to the memory location that helps us to store some form of data and retrieves it when required. It allows us to refer to memory location without having to memorize the memory address. A variable name can be used in expressions as a substitute in place of the value it s
3 min read
Constants in C
The constants in C are the read-only variables whose values cannot be modified once they are declared in the C program. The type of constant can be an integer constant, a floating pointer constant, a string constant, or a character constant. In C language, the const keyword is used to define the con
6 min read
Const Qualifier in C
The qualifier const can be applied to the declaration of any variable to specify that its value will not be changed (which depends upon where const variables are stored, we may change the value of the const variable by using a pointer). The result is implementation-defined if an attempt is made to c
6 min read
Different ways to declare variable as constant in C
There are many different ways to make the variable as constant in C. Some of the popular ones are: Using const KeywordUsing MacrosUsing enum Keyword1. Using const KeywordThe const keyword specifies that a variable or object value is constant and can't be modified at the compilation time. Syntaxconst
2 min read
Scope rules in C
The scope of a variable in C is the block or the region in the program where a variable is declared, defined, and used. Outside this region, we cannot access the variable, and it is treated as an undeclared identifier. The scope is the area under which a variable is visible.The scope of an identifie
6 min read
Internal Linkage and External Linkage in C
In C, linkage is a concept that describes how names/identifiers can or cannot refer to the same entity throughout the whole program or a single translation unit. The above sounds similar to scope, but it is not so. To understand what the above means, let us dig deeper into the compilation process. B
5 min read
Global Variables in C
Prerequisite: Variables in C In a programming language, each variable has a particular scope attached to them. The scope is either local or global. This article will go through global variables, their advantages, and their properties. The Declaration of a global variable is very similar to that of a
3 min read
C Data Types
Data Types in C
Each variable in C has an associated data type. It specifies the type of data that the variable can store like integer, character, floating, double, etc. Each data type requires different amounts of memory and has some specific operations which can be performed over it. The data types in C can be cl
7 min read
Literals in C
In C, Literals are the constant values that are assigned to the variables. Literals represent fixed values that cannot be modified. Literals contain memory but they do not have references as variables. Generally, both terms, constants, and literals are used interchangeably. For example, âconst int =
4 min read
Escape Sequence in C
The escape sequence in C is the characters or the sequence of characters that can be used inside the string literal. The purpose of the escape sequence is to represent the characters that cannot be used normally using the keyboard. Some escape sequence characters are the part of ASCII charset but so
5 min read
bool in C
The bool in C is a fundamental data type in most that can hold one of two values: true or false. It is used to represent logical values and is commonly used in programming to control the flow of execution in decision-making statements such as if-else statements, while loops, and for loops. In this a
6 min read
Integer Promotions in C
Some data types like char , short int take less number of bytes than int, these data types are automatically promoted to int or unsigned int when an operation is performed on them. This is called integer promotion. For example no arithmetic calculation happens on smaller types like char, short and e
2 min read
Character Arithmetic in C
As already known character range is between -128 to 127 or 0 to 255. This point has to be kept in mind while doing character arithmetic. What is Character Arithmetic?Character arithmetic is used to implement arithmetic operations like addition, subtraction, multiplication, and division on characters
2 min read
Type Conversion in C
In C, type conversion refers to the process of converting one data type to another. It can be done automatically by the compiler or manually by the programmer. The type conversion is only performed to those data types where conversion is possible. Let's take a look at an example: [GFGTABS] C #includ
4 min read
C Input/Output
Basic Input and Output in C
In C programming, input and output operations refer to reading data from external sources and writing data to external destinations outside the program. C provides a standard set of functions to handle input from the user and output to the screen or to files. These functions are part of the standard
2 min read
Format Specifiers in C
The format specifier in C is used to tell the compiler about the type of data to be printed or scanned in input and output operations. They always start with a % symbol and are used in the formatted string in functions like printf(), scanf, sprintf(), etc. The C language provides a number of format
6 min read
printf in C
In C language, printf() function is used to print formatted output to the standard output stdout (which is generally the console screen). The printf function is the most used output function in C and it allows formatting the output in many ways. Example: [GFGTABS] C #include <stdio.h> int main
6 min read
scanf in C
In C programming language, scanf is a function that stands for Scan Formatted String. It is used to read data from stdin (standard input stream i.e. usually keyboard) and then writes the result into the given arguments. It accepts character, string, and numeric data from the user using standard inpu
2 min read
Scansets in C
scanf family functions support scanset specifiers which are represented by %[]. Inside scanset, we can specify single character or range of characters. While processing scanset, scanf will process only those characters which are part of scanset. We can define scanset by putting characters inside squ
2 min read
Formatted and Unformatted Input/Output functions in C with Examples
This article focuses on discussing the following topics in detail- Formatted I/O Functions.Unformatted I/O Functions.Formatted I/O Functions vs Unformatted I/O Functions.Formatted I/O Functions Formatted I/O functions are used to take various inputs from the user and display multiple outputs to the
9 min read
C Operators
Operators in C
In C language, operators are symbols that represent operations to be performed on one or more operands. They are the basic components of the C programming. In this article, we will learn about all the built-in operators in C with examples. What is a C Operator?An operator in C can be defined as the
14 min read
Arithmetic Operators in C
Arithmetic operators are the type of operators used to perform basic math operations like addition, subtraction, and multiplication. Let's take a look at an example: [GFGTABS] C #include <stdio.h> int main() { // Calculate the area of the triangle int sum = 10 + 20; printf("%d", sum)
5 min read
Unary operators in C
Unary operators are the operators that perform operations on a single operand to produce a new value. Types of unary operatorsTypes of unary operators are mentioned below: Unary minus ( - )Increment ( ++ )Decrement ( -- )NOT ( ! )Addressof operator ( & )sizeof()1. Unary MinusThe minus operator (
4 min read
Relational Operators in C
In C, relational operators are the symbols that are used for comparison between two values to understand the type of relationship a pair of numbers shares. The result that we get after the relational operation is a boolean value, that tells whether the comparison is true or false. Relational operato
4 min read
Bitwise Operators in C
In C, the following 6 operators are bitwise operators (also known as bit operators as they work at the bit-level). They are used to perform bitwise operations in C. The & (bitwise AND) in C takes two numbers as operands and does AND on every bit of two numbers. The result of AND is 1 only if bot
7 min read
C Logical Operators
Logical operators in C are used to combine multiple conditions/constraints. Logical Operators returns either 0 or 1, it depends on whether the expression result is true or false. In C programming for decision-making, we use logical operators. We have 3 logical operators in the C language: Logical AN
6 min read
Assignment Operators in C
Assignment operators are used for assigning value to a variable. The left side operand of the assignment operator is a variable and right side operand of the assignment operator is a value. The value on the right side must be of the same data-type of the variable on the left side otherwise the compi
3 min read
Increment and Decrement Operators in C
The increment ( ++ ) and decrement ( -- ) operators in C are unary operators for incrementing and decrementing the numeric values by 1 respectively. The incrementation and decrementation are one of the most frequently used operations in programming for looping, array traversal, pointer arithmetic, a
4 min read
Conditional or Ternary Operator (?:) in C
The conditional operator in C is kind of similar to the if-else statement as it follows the same algorithm as of if-else statement but the conditional operator takes less space and helps to write the if-else statements in the shortest way possible. It is also known as the ternary operator in C as it
3 min read
sizeof operator in C
Sizeof is a much-used operator in the C. It is a compile-time unary operator which can be used to compute the size of its operand. The result of sizeof is of the unsigned integral type which is usually denoted by size_t. sizeof can be applied to any data type, including primitive types such as integ
3 min read
Operator Precedence and Associativity in C
Operator precedence and associativity are rules that decide the order in which parts of an expression are calculated. Precedence tells us which operators should be evaluated first, while associativity determines the direction (left to right or right to left) in which operators with the same preceden
8 min read
C Control Statements Decision-Making
Decision Making in C (if , if..else, Nested if, if-else-if )
The conditional statements (also known as decision control structures) such as if, if else, switch, etc. are used for decision-making purposes in C programs. They are also known as Decision-Making Statements and are used to evaluate one or more conditions and make the decision whether to execute a s
11 min read
C - if Statement
The if in C is the simplest decision-making statement. It consists of the test condition and a block of code that is executed if and only if the given condition is true. Otherwise, it is skipped from execution. Let's take a look at an example: [GFGTABS] C #include <stdio.h> int main() { int n
4 min read
C if else Statement
The if else in C is an extension of the if statement which not only allows the program to execute one block of code if a condition is true, but also a different block if the condition is false. This enables making decisions with two possible outcomes. Let's take a look at an example: [GFGTABS] C #in
3 min read
C if else if ladder
In C, if else if ladder is an extension of if else statement used to test a series of conditions sequentially, executing the code for the first true condition. A condition is checked only if all previous ones are false. Once a condition is true, its code block executes, and the ladder ends. Example:
3 min read
Switch Statement in C
In C, switch statement is a control flow structure that allows you to execute one of many code blocks based on the value of an expression. It is often used in place of if-else ladder when there are multiple conditional codes. Example: [GFGTABS] C #include <stdio.h> int main() { // Switch varia
6 min read
Using Range in switch Case in C
You all are familiar with switch case in C, but did you know you can use a range of numbers instead of a single number or character in the case statement? Range in switch case can be useful when we want to run the same set of statements for a range of numbers so that we do not have to write cases se
2 min read
C - Loops
Loops in programming are used to repeat a block of code until the specified condition is met. A loop statement allows programmers to execute a statement or group of statements multiple times without repetition of code. [GFGTABS] C // C program to illustrate need of loops #include <stdio.h> int
6 min read
C for Loop
In C programming, the for loop is used to repeatedly execute a block of code as many times as instructed. It uses a variable (loop variable) whose value is used to decide the number of repetitions. It is generally used when we know how many times we want to repeat the code. Let's take a look at an e
4 min read
while Loop in C
The while loop in C allows a block of code to be executed repeatedly as long as a given condition remains true. It is often used when we want to repeat a block of code till some condition is satisfied. Let's take a look at an example: [GFGTABS] C #include <stdio.h> int main() { int i = 1; // C
5 min read
do...while Loop in C
The do...while loop is a type of loop in C that executes a block of code until the given condition is satisfied. The feature of do while loops is that unlike the while loop, which checks the condition before executing the loop, the do...while loop ensures that the code inside the loop is executed at
4 min read
For vs. While
In C, oops are the fundamental part of language that are used to repeat a block of code multiple times. The two most commonly used loops are the for loop and the while loop. Although they achieve the same result, their structure, use cases, and flexibility differ. The below table highlights some pri
4 min read
Continue Statement in C
The continue statement in C is a jump statement used to skip the current iteration of a loop and continue with the next iteration. It is used inside loops (for, while, or do-while) along with the conditional statements to bypass the remaining statements in the current iteration and move on to next i
4 min read
Break Statement in C
The break in C is a loop control statement that breaks out of the loop when encountered. It can be used inside loops or switch statements to bring the control out of the block. The break statement can only break out of a single loop at a time. Let's take a look at an example: [GFGTABS] C #include
6 min read
goto Statement in C
The goto statement in C allows the program to jump to some part of the code, giving you more control over its execution. While it can be useful in certain situations, like error handling or exiting complex loops, it's generally not recommended because it can make the code harder to read and maintain
4 min read
C Functions
C Functions
A function in C is a set of statements that when called perform some specific tasks. It is the basic building block of a C program that provides modularity and code reusability. The programming statements of a function are enclosed within { } braces, having certain meanings and performing certain op
10 min read
User-Defined Function in C
A user-defined function is a type of function in C language that is defined by the user himself to perform some specific task. It provides code reusability and modularity to our program. User-defined functions are different from built-in functions as their working is specified by the user and no hea
6 min read
Parameter Passing Techniques in C
In C, there are different ways in which parameter data can be passed into and out of methods and functions. Let us assume that a function B() is called from another function A(). In this case, A is called the "caller function" and B is called the "called function or callee function". Also, the argum
5 min read
Function Prototype in C
In C, a function prototype is a statement that tells the compiler about the functionâs name, its return type, numbers, and data types of its parameters. Using this information, the compiler cross-checks function parameters and their data type with function definition and function call. For example,
6 min read
How can I return multiple values from a function?
In C programming, a function can return only one value directly. However, C also provides several indirect methods in to return multiple values from a function. In this article, we will learn the different ways to return multiple values from a function in C. The most straightforward method to return
3 min read
main Function in C
The main function is the entry point of a C program. It is a user-defined function where the execution of a program starts. Every C program must contain, and its return value typically indicates the success or failure of the program. In this article, we will learn more about the main function in C.
5 min read
Implicit Return Type int in C
In C, every function has a return type that indicates the type of value it will return, and it is defined at the time of function declaration or definition. But in C language, it is possible to define functions without mentioning the return type and by default, int is implicitly assumed that the ret
2 min read
Callbacks in C
A callback is any executable code that is passed as an argument to another code, which is expected to call back (execute) the argument at a given time. In simple terms, a callback is the process of passing a function (executable code) to anther function as argument and then it is called by the passe
4 min read
Nested functions in C
Some programmer thinks that defining a function inside an another function is known as "nested function". But the reality is that it is not a nested function, it is treated as lexical scoping. Lexical scoping is not valid in C because the compiler cant reach/find the correct memory location of the i
2 min read
Variadic Functions in C
In C, variadic functions are functions that can take a variable number of arguments. This feature is useful when the number of arguments for a function is unknown. It takes one fixed argument and then any number of arguments can be passed. Let's take a look at an example: [GFGTABS] C #include <st
5 min read
_Noreturn function specifier in C
In C, the _Noreturn specifier is used to indicate that a function does not return a value. It tells the compiler that the function will either exit the program or enter an infinite loop, so it will never return control to the calling function. This helps the compiler to optimize code and issue warni
2 min read
Predefined Identifier __func__ in C
Before we start discussing __func__, let us write some code snippets and anticipate the output: C/C++ Code // C program to demonstrate working of a // Predefined Identifier __func__ #include <stdio.h> int main() { // %s indicates that the program will read strings printf("%s", __func
2 min read
C Library math.h Functions
The math.h header defines various C mathematical functions and one macro. All the functions available in this library take double as an argument and return double as the result. Let us discuss some important C math functions one by one. C Math Functions1. double ceil (double x) The C library functio
6 min read
C Arrays & Strings
C Arrays
Array in C is one of the most used data structures in C programming. It is a simple and fast way of storing multiple values under a single name. In this article, we will study the different aspects of array in C language such as array declaration, definition, initialization, types of arrays, array s
15+ min read
Properties of Array in C
An array in C is a fixed-size homogeneous collection of elements stored at a contiguous memory location. It is a derived data type in C that can store elements of different data types such as int, char, struct, etc. It is one of the most popular data types widely used by programmers to solve differe
8 min read
Multidimensional Arrays in C - 2D and 3D Arrays
Prerequisite: Arrays in C A multi-dimensional array can be defined as an array that has more than one dimension. Having more than one dimension means that it can grow in multiple directions. Some popular multidimensional arrays are 2D arrays and 3D arrays. In this article, we will learn about multid
10 min read
Initialization of Multidimensional Array in C
In C, multidimensional arrays are the arrays that contain more than one dimensions. These arrays are useful when we need to store data in a table or matrix-like structure. In this article, we will learn the different methods to initialize a multidimensional array in C. The easiest method for initial
4 min read
Pass Array to Functions in C
Passing an array to a function allows the function to directly access and modify the original array. In this article, we will learn how to pass arrays to functions in C. In C, arrays are always passed to function as pointers. They cannot be passed by value because of the array decay due to which, wh
3 min read
How to pass a 2D array as a parameter in C?
This post is an extension of How to dynamically allocate a 2D array in C? A one dimensional array can be easily passed as a pointer, but syntax for passing a 2D array to a function can be difficult to remember. One important thing for passing multidimensional arrays is, first array dimension does no
4 min read
What are the data types for which it is not possible to create an array?
In C, an array is a collection of variables of the same data type, stored in contiguous memory locations. Arrays can store data of primitive types like integers, characters, and floats, as well as user-defined types like structures. However, there are certain data types for which arrays cannot be di
2 min read
How to pass an array by value in C ?
In C programming, arrays are always passed as pointers to the function. There are no direct ways to pass the array by value. However, there is trick that allows you to simulate the passing of array by value by enclosing it inside a structure and then passing that structure by value. This will also p
2 min read
Strings in C
A String in C programming is a sequence of characters terminated with a null character '\0'. The C String is stored as an array of characters. The difference between a character array and a C string is that the string in C is terminated with a unique character '\0'. C String Declaration SyntaxDeclar
8 min read
Array of Strings in C
In C, an array of strings is a 2D array where each row contains a sequence of characters terminated by a '\0' NULL character (strings). It is used to store multiple strings in a single array. Let's take a look at an example: [GFGTABS] C #include <stdio.h> int main() { // Creating array of stri
3 min read
What is the difference between single quoted and double quoted declaration of char array?
In C programming, the way we declare and initialize a char array can differ based on whether we want to use a sequence of characters and strings. They are basically same with difference only of a '\0' NULL character. Double quotes automatically include the null terminator, making the array a string
2 min read
C String Functions
The C string functions are built-in functions that can be used for various operations and manipulations on strings. These string functions make it easier to perform tasks such as string copy, concatenation, comparison, length, etc. The <string.h> header file contains these string functions. Th
6 min read
C Pointers
C Pointers
A pointer is a variable that stores the memory address of another variable. Instead of holding a direct value, it holds the address where the value is stored in memory. There are 2 important operators that we will use in pointers concepts i.e. Dereferencing operator(*) used to declare pointer variab
10 min read
Pointer Arithmetics in C with Examples
Pointer Arithmetic is the set of valid arithmetic operations that can be performed on pointers. The pointer variables store the memory address of another variable. It doesn't store any value. Hence, there are only a few operations that are allowed to perform on Pointers in C language. The C pointer
10 min read
C - Pointer to Pointer (Double Pointer)
In C, double pointers are those pointers which stores the address of another pointer. The first pointer is used to store the address of the variable, and the second pointer is used to store the address of the first pointer. That is why they are also known as a pointer to pointer. Let's take a look a
5 min read
Function Pointer in C
In C, a function pointer is a type of pointer that stores the address of a function, allowing functions to be passed as arguments and invoked dynamically. It is useful in techniques such as callback functions, event-driven programs, and polymorphism (a concept where a function or operator behaves di
6 min read
How to Declare a Pointer to a Function?
A pointer to a function is similar to a pointer to a variable. However, instead of pointing to a variable, it points to the address of a function. This allows the function to be called indirectly, which is useful in situations like callback functions or event-driven programming. In this article, we
2 min read
Pointer to an Array | Array Pointer
A pointer to an array is a pointer that points to the whole array instead of the first element of the array. It considers the whole array as a single unit instead of it being a collection of given elements. Consider the following example: [GFGTABS] C #include<stdio.h> int main() { int arr[5] =
5 min read
Difference between constant pointer, pointers to constant, and constant pointers to constants
In this article, we will discuss the differences between constant pointer, pointers to constant & constant pointers to constants. Pointers are the variables that hold the address of some other variables, constants, or functions. There are several ways to qualify pointers using const. Pointers to
3 min read
Pointer vs Array in C
Most of the time, pointer and array accesses can be treated as acting the same, the major exceptions being: 1. the sizeof operator sizeof(array) returns the amount of memory used by all elements in the array sizeof(pointer) only returns the amount of memory used by the pointer variable itself 2. the
1 min read
Dangling, Void , Null and Wild Pointers in C
In C programming pointers are used to manipulate memory addresses, to store the address of some variable or memory location. But certain situations and characteristics related to pointers become challenging in terms of memory safety and program behavior these include Dangling (when pointing to deall
6 min read
Near, Far and Huge Pointers in C
In older times, the intel processors had 16-bit registers, but the address bus was 20-bits wide. Due to this, CPU registers were not able to hold the entire address at once. As a solution, the memory was divided into segments of 64 kB size, and the near pointers, far pointers, and huge pointers were
4 min read
restrict Keyword in C
The restrict keyword is a type qualifier that was introduced in the C99 standard. It is used to tell the compiler that a pointer is the only reference or access point to the memory it points to, allowing the compiler to make optimizations based on that information. Let's take a look at an example: [
3 min read