How to Generate Pattern Password in Android?
Last Updated :
01 Aug, 2024
Pattern Password for the device is one of the necessities to keep our device private and protected. Nowadays in most apps, we get to see this password applied in many applications such as Mutual Funds or Stock Market apps to keep our financial details private. In this article, we are going to see how to implement pattern passwords in our Android app. A sample GIF is given below to get an idea about what we are going to do in this article. Note that we are going to implement this project using the Java language.
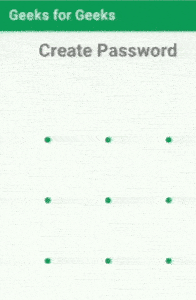
pattern password
Applications of Pattern Password
- Use to protect our private details and personal information in an Android app.
- It becomes very convenient to use pattern passwords instead of pins.
- It works as an app Lock to our app on our device.
Attributes of Pattern Password
Attributes | Description |
---|
layout_width | To display the width of the dots. |
layout_height | To display the height of the dots. |
correctStateColor | To display Dots color. |
Step by Step Implementation
Step 1: Create a New Project
To create a new project in Android Studio please refer to How to Create/Start a New Project in Android Studio. Note that select Java as the programming language.
Step 2: Add dependency of Pattern Password library in build.gradle file
Then Navigate to gradle scripts and then to build.gradle(Module) level. Add below line in build.gradle file in the dependencies section.
implementation ‘com.andrognito.patternlockview:patternlockview:1.0.0’
now click on Sync now it will sync your all files in build.gradle().
Step 3: Create a Loading Screen in your activity_main.xml file
Navigate to the app > res > layout to open the activity_main.xml file. Below is the code for the activity_main.xml file.
XML
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<!--Text view for giving loading-->
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerInParent="true"
android:text="Loading...." />
</RelativeLayout>
Step 4: Working with the MainActivity.java file
Go to the MainActivity.java file and refer to the following code. Below is the code for the MainActivity.java file. Comments are added inside the code to understand the code in more detail.
Java
import android.content.Intent;
import android.content.SharedPreferences;
import android.os.Bundle;
import android.os.Handler;
import androidx.appcompat.app.AppCompatActivity;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Handler handler = new Handler();
handler.postDelayed(new Runnable() {
@Override
public void run() {
// loading is given
SharedPreferences sharedPreferences = getSharedPreferences("PREFS", 0);
String password = sharedPreferences.getString("password", "0");
if (password.equals("0")) {
// Intent to navigate to Create Password Screen
Intent intent = new Intent(getApplicationContext(), CreatePasswordActivity.class);
startActivity(intent);
finish();
} else {
// Intent to navigate to Input Password Screen
Intent intent = new Intent(getApplicationContext(), InputPasswordActivity.class);
startActivity(intent);
finish();
}
}
}, 2000);
}
}
Step 5: Create a new empty Activity and name it as CreatePasswordActivity
Go to the app > java > Your app’s package name > right-click > New > Activity > Empty Activity and name the activity as CreatePasswordActivity.
Working with the activity_create_password.xml file:
Go to the activity_create_password.xml file and refer to the following code. Below is the code for the activity_create_password.xml file. In this file, you will show dots to create a pattern password as given below.
XML
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent">
<!--Dots to create pattern pass word-->
<com.andrognito.patternlockview.PatternLockView
android:id="@+id/pattern_lock_view"
android:layout_width="300dp"
android:layout_height="300dp"
android:layout_centerInParent="true"
app:correctStateColor="@color/purple_200"
app:normalStateColor="@color/purple_200"
app:wrongStateColor="@color/teal_700" />
<!--Text View to display title-->
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerHorizontal="true"
android:layout_marginTop="10dp"
android:text="Create Password"
android:textSize="30dp"
android:textStyle="bold" />
</RelativeLayout>
Working with the CreatePasswordActivity.java file:
Go to the CreatePasswordActivity.java file and refer to the following code. Below is the code for the CreatePasswordActivity.java file. Comments are added inside the code to understand the code in more detail.
Java
import android.content.Intent;
import android.content.SharedPreferences;
import android.os.Bundle;
import androidx.appcompat.app.AppCompatActivity;
import com.andrognito.patternlockview.PatternLockView;
import com.andrognito.patternlockview.listener.PatternLockViewListener;
import com.andrognito.patternlockview.utils.PatternLockUtils;
import java.util.List;
public class CreatePasswordActivity extends AppCompatActivity {
// Initialize pattern lock view
PatternLockView mPatternLockView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_create_password);
mPatternLockView = (PatternLockView) findViewById(R.id.pattern_lock_view);
mPatternLockView.addPatternLockListener(new PatternLockViewListener() {
@Override
public void onStarted() {
}
@Override
public void onProgress(List<PatternLockView.Dot> progressPattern) {
}
@Override
public void onComplete(List<PatternLockView.Dot> pattern) {
// Shared Preferences to save state
SharedPreferences sharedPreferences = getSharedPreferences("PREFS", 0);
SharedPreferences.Editor editor = sharedPreferences.edit();
editor.putString("password", PatternLockUtils.patternToString(mPatternLockView, pattern));
editor.apply();
// Intent to navigate to home screen when password added is true
Intent intent = new Intent(getApplicationContext(), ProgramActivity.class);
startActivity(intent);
finish();
}
@Override
public void onCleared() {
}
});
}
}
Step 6: Similarly Create a new empty Activity and name it as InputPasswordActivity
Go to the app > java > Your app’s package name > right-click > New > Activity > Empty Activity and name the activity as InputPasswordActivity.
Working with the activity_Input_Password.xml file:
Go to the activity_Input_Password.xml file and refer to the following code. Below is the code for the activity_Input_Password.xml file. In this file, you will draw your pattern to navigate to the home screen when the user opened the app for the second time after installing.
XML
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent">
<!--Dots to input password-->
<com.andrognito.patternlockview.PatternLockView
android:id="@+id/pattern_lock_view"
android:layout_width="300dp"
android:layout_height="300dp"
android:layout_centerInParent="true"
app:correctStateColor="@color/purple_200"
app:normalStateColor="@color/purple_200"
app:wrongStateColor="@color/teal_700" />
<!--Text View to display title-->
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerHorizontal="true"
android:layout_marginTop="10dp"
android:text="Input Password"
android:textSize="30dp"
android:textStyle="bold" />
</RelativeLayout>
Working with the InputPasswordActivity.java file:
Go to the InputPasswordActivity.java file and refer to the following code. Below is the code for the InputPasswordActivity.java file. Comments are added inside the code to understand the code in more detail.
Java
import android.content.Intent;
import android.content.SharedPreferences;
import android.os.Bundle;
import android.widget.Toast;
import androidx.appcompat.app.AppCompatActivity;
import com.andrognito.patternlockview.PatternLockView;
import com.andrognito.patternlockview.listener.PatternLockViewListener;
import com.andrognito.patternlockview.utils.PatternLockUtils;
import java.util.List;
public class InputPasswordActivity extends AppCompatActivity {
PatternLockView mPatternLockView;
String password;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_input_password);
// shared preference when user comes second time to the app
SharedPreferences sharedPreferences = getSharedPreferences("PREFS", 0);
password = sharedPreferences.getString("password", "0");
mPatternLockView = (PatternLockView) findViewById(R.id.pattern_lock_view);
mPatternLockView.addPatternLockListener(new PatternLockViewListener() {
@Override
public void onStarted() {
}
@Override
public void onProgress(List<PatternLockView.Dot> progressPattern) {
}
@Override
public void onComplete(List<PatternLockView.Dot> pattern) {
// if drawn pattern is equal to created pattern you will navigate to home screen
if (password.equals(PatternLockUtils.patternToString(mPatternLockView, pattern))) {
Intent intent = new Intent(getApplicationContext(), ProgramActivity.class);
startActivity(intent);
finish();
} else {
// other wise you will get error wrong password
Toast.makeText(InputPasswordActivity.this, "Wrong Password", Toast.LENGTH_SHORT).show();
mPatternLockView.clearPattern();
}
}
@Override
public void onCleared() {
}
});
}
}
Step 7: Similarly, Create a new empty Activity and name it as ProgramActivity
Go to the app > java > Your app’s package name > right-click > New > Activity > Empty Activity and name the activity as ProgramActivity.
Working with the activity_program.xml file:
Go to the activity_program.xml file and refer to the following code. Below is the code for the activity_program.xml file. In this file, we will create Home Screen to navigate the user to the main screen. Where you will get to see the text message.
XML
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent">
<!--Text Message to display-->
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerInParent="true"
android:text="Welcome to Geeks to Geeks"
android:textSize="20dp"
tools:layout_editor_absoluteX="163dp"
tools:layout_editor_absoluteY="334dp" />
</RelativeLayout>
Now click on the run option it will take some time to build Gradle. After that, you will get output on your device as given below.
Output:
Similar Reads
How to Generate Pattern Password in Android?
Pattern Password for the device is one of the necessities to keep our device private and protected. Nowadays in most apps, we get to see this password applied in many applications such as Mutual Funds or Stock Market apps to keep our financial details private. In this article, we are going to see ho
6 min read
How to Generate QR Code in Android?
QR codes are used in many apps to display data in machine-readable form. These codes are used to represent data in a secure manner that is readable only by machines and not by humans. We have seen many apps that provide QR codes and we can scan those QR codes with our mobile device. In this article,
3 min read
How to Change Password of User in Android using Firebase?
In many apps, we got a feature to login using our email and password. Sometimes it happens that we forget the password and most of the time there reset our password. Here we are going to implement the same feature to Reset our password using Firebase Authentication. You may refer to the following ar
3 min read
Random password generator in C
In this article, we will discuss how to generate a random password of a given length consists of any characters. Approach: The below-given program involves basic concepts like variables, data types, array, loop, etc.Follow the below steps to solve this problem:Take the length of the password and dec
2 min read
How to Generate Barcode in Android?
Barcodes are the graphical representation of data that enables effortless scanning. When we aim to incorporate this functionality for various purposes in Android applications it becomes crucial to understand the underlying principles and techniques involved in generating barcodes effectively. In thi
3 min read
How to Toggle Password Visibility in Android?
As we know toggling means switching between the two different options by pressing a single button. So In this article, we will be seeing how to change the password visibility by pressing a single button (here it would be the eye button), ie with an input of password type, we can also enable an icon,
3 min read
How to Validate Password from Text Input in Android?
Whenever we type the password in most social sites or any web and android application, We get an alert to add a minimum of one lowercase alphabet, minimum one uppercase alphabet, minimum one numerical digit and length of password must be greater than or equal to 8. So In this article, we are going t
3 min read
How to Generate a Random Number From a Range in Android?
In Android, there are multiple libraries that provide mathematical and logical functions to the developers to create meaningful applications. One such function is the random function that generates a random value. However, we can customize this function to not just find a random value but find it fr
3 min read
How to Generate SHA1, MD5, and SHA-256 Keys in Android Studio?
SHA1, MD5, and SHA-256 are cryptographic functions that will convert your input to 160 bit (20 bytes) value. It is a secure key that is used to store very important data. In Android SHA1, MD5 and SA-256 keys are very important. If you want to add external APIs from Google such as Maps and other exte
3 min read
How to Validate a Password using Regular Expressions in Android?
Regular Expression basically defines a search pattern, pattern matching, or string matching. It is present in java.util.regex package. Java Regex API provides 1 interface and 3 classes. They are the following: MatchResult InterfaceMatcher classPattern classPatternSyntaxException class Pattern p = Pa
4 min read