Memento Method Design Pattern in Python
The Memento Design Pattern is a behavioral design pattern that allows you to capture and restore an object’s state without revealing its internal structure. This pattern is particularly useful in implementing undo mechanisms. In this article, we will explore the Memento Method Design Pattern in Python, providing practical examples and use cases to help you understand how to implement and utilize this pattern effectively.
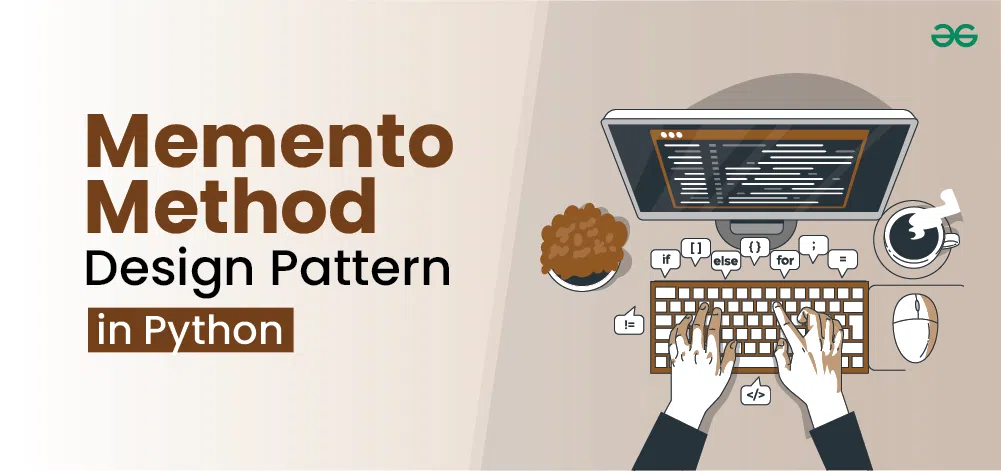
Memento Method Design Pattern in Python
Important Topics for Memento Method Design Pattern in Python
- What is the Memento Method Design Pattern?
- Components of the Memento Method Design Pattern
- Class Diagram of Memento Method Design Pattern
- Example implementation of the Memento Method Design Pattern in Python
- When to use Memento Method Design Pattern in Python?
- When not to use Memento Method Design Pattern in Python?
What is the Memento Method Design Pattern?
The Memento Design Pattern is a behavioral design pattern used in software development to capture and externalize an object’s internal state so that it can be restored later without violating encapsulation. The Memento pattern is useful for implementing features like undo/redo operations, state persistence, and checkpoints in applications, allowing the system to revert to a previous state when needed.
Components of the Memento Method Design Pattern
The Memento Method Design Pattern comprises three main components:
1. Originator
- The object whose internal state is to be saved and restored.
- It creates a Memento containing a snapshot of its current state and can use the Memento to restore its state later.
- Example: In a text editor, the Originator could be the document being edited.
2. Memento
- The object that stores the internal state of the Originator.
- It is a snapshot of the Originator’s state at a particular point in time.
- Mementos are usually immutable to ensure the state cannot be modified once it is captured.
- Example: In a text editor, the Memento could store the content of the document at a specific time.
3. Caretaker
- The object responsible for managing the Memento.
- It requests a Memento from the Originator, holds it, and passes it back to the Originator when needed for state restoration.
- The Caretaker does not modify or inspect the contents of the Memento.
- Example: In a text editor, the Caretaker could be the undo manager that keeps a stack of Mementos for undo/redo operations.
These components work together to provide a mechanism for saving and restoring an object’s state without exposing its internal details, thus maintaining encapsulation and ensuring state management is handled cleanly and efficiently.
Class Diagram of Memento Method Design Pattern

Class Diagram of Memento Method Design Pattern
- The caretaker calls the createMemento() method on the originator asking the originator to pass it a memento object.
- At this point the originator creates a memento object saving its internal state and passes the memento to the caretaker.
- The caretaker maintains the memento object and performs the operation. In case of the need to undo the operation, the caretaker calls the setMemento() method on the originator passing the maintained memento object.
- The originator would accept the memento, using it to restore its previous state.
Example implementation of the Memento Method Design Pattern in Python
Problem Statement:
Consider a text editor application where users can type and edit text. The application needs to support undo and redo operations so that users can revert to previous versions of their text or redo actions they have undone. Implementing these features requires saving and restoring the state of the text document at different points in time.
How Memento Method Design Pattern helps to solve this problem?
The Memento Design Pattern can be used to capture the state of the text document (i.e., the text content) at different points in time, allowing users to undo or redo changes. The pattern involves:
- The Originator (the text editor) which creates and restores Mementos.
- The Memento which stores the state of the text.
- The Caretaker which manages the Mementos (e.g., keeping track of undo and redo operations).

Class Diagram of Memento Method Design Pattern for above example
Below is the implementation of the above solution:
1. Originator
The Originator class represents the text editor that can create and restore Mementos.
class Originator:
def __init__(self, text):
self.text = text
def set_text(self, text):
self.text = text
def create_memento(self):
return Memento(self.text)
def restore_memento(self, memento):
self.text = memento.get_state()
def __str__(self):
return self.text
2. Memento
The Memento class stores the state of the Originator.
class Memento:
def __init__(self, state):
self._state = state
def get_state(self):
return self._state
3. Caretaker
The Caretaker class manages the Mementos, allowing undo and redo operations.
class Caretaker:
def __init__(self):
self._mementos = []
self._current_index = -1
def save(self, memento):
# Remove future states if any
if self._current_index + 1 < len(self._mementos):
self._mementos = self._mementos[:self._current_index + 1]
self._mementos.append(memento)
self._current_index += 1
def undo(self):
if self._current_index > 0:
self._current_index -= 1
return self._mementos[self._current_index]
return None
def redo(self):
if self._current_index + 1 < len(self._mementos):
self._current_index += 1
return self._mementos[self._current_index]
return None
Below is the complete combined code discussed above:
class Originator:
def __init__(self, text):
self.text = text
def set_text(self, text):
self.text = text
def create_memento(self):
return Memento(self.text)
def restore_memento(self, memento):
self.text = memento.get_state()
def __str__(self):
return self.text
class Memento:
def __init__(self, state):
self._state = state
def get_state(self):
return self._state
class Caretaker:
def __init__(self):
self._mementos = []
self._current_index = -1
def save(self, memento):
# Remove future states if any
if self._current_index + 1 < len(self._mementos):
self._mementos = self._mementos[:self._current_index + 1]
self._mementos.append(memento)
self._current_index += 1
def undo(self):
if self._current_index > 0:
self._current_index -= 1
return self._mementos[self._current_index]
return None
def redo(self):
if self._current_index + 1 < len(self._mementos):
self._current_index += 1
return self._mementos[self._current_index]
return None
# Example usage
if __name__ == "__main__":
# Create an Originator (Text Editor)
editor = Originator("Initial Text")
# Create a Caretaker
caretaker = Caretaker()
# Save the initial state
caretaker.save(editor.create_memento())
# Make changes and save the state
editor.set_text("First Edit")
caretaker.save(editor.create_memento())
editor.set_text("Second Edit")
caretaker.save(editor.create_memento())
# Perform undo operations
print("Current State:", editor) # Output: "Second Edit"
memento = caretaker.undo()
if memento:
editor.restore_memento(memento)
print("After Undo:", editor) # Output: "First Edit"
# Perform redo operations
memento = caretaker.redo()
if memento:
editor.restore_memento(memento)
print("After Redo:", editor) # Output: "Second Edit"
Below is the explanation of the above code:
- Originator:
- The
Originator
class represents the text editor with methods to set text, create Mementos, and restore from Mementos. - It maintains the current state of the text and allows for the creation and restoration of Mementos.
- The
- Memento:
- The
Memento
class holds a snapshot of the Originator’s state. It provides a method to retrieve the state but does not allow modifications.
- The
- Caretaker:
- The
Caretaker
class manages a list of Mementos, enabling undo and redo functionality. - It saves Mementos, and can undo or redo changes by moving the index in the list of Mementos.
- The
By using the Memento Design Pattern, the text editor application can efficiently manage state changes, allowing users to revert to previous text versions without exposing the internal details of the text management system.
When to use Memento Method Design Pattern in Python?
The Memento Design Pattern is particularly useful in scenarios where you need to capture and restore the state of an object without exposing its internal details. Here’s when you should consider using the Memento Pattern:
- Undo/Redo Functionality:
- Use Case: When implementing features like undo and redo in applications such as text editors or graphic design tools.
- Example: A text editor where users need to revert to previous versions of their document or redo actions that were undone.
- Snapshot Management:
- Use Case: When you need to save and restore the state of an object at specific points in time, allowing for historical states to be revisited.
- Example: A game where you want to save the state of the game (e.g., player position, scores) at various checkpoints.
- State Preservation Across Transactions:
- Use Case: When you need to preserve the state of an object across multiple transactions or operations, ensuring that the state can be recovered if necessary.
- Example: An online shopping cart that allows users to revert to a previous state if they navigate away from the cart and come back later.
- Encapsulation of State Management:
- Use Case: When you want to encapsulate the state management of an object to avoid exposing its internal details and ensure that the state management is handled in a controlled manner.
- Example: A drawing application where the state of the canvas (e.g., drawings, colors) needs to be managed without exposing the internal structure of the drawing objects.
- Temporary State Storage:
- Use Case: When you need to temporarily store the state of an object for a specific duration and restore it later without affecting the main object’s state.
- Example: A configuration management system where temporary configurations can be saved and restored as needed.
When not to use Memento Method Design Pattern in Python?
The Memento Method Design Pattern, while useful in many scenarios, is not always the best choice. Here are situations where you might want to avoid using the Memento Pattern:
- Simple State Management:
- Reason: If the state of the object is simple and does not require frequent saving or restoration, the overhead of implementing the Memento Pattern might be unnecessary.
- Example: A basic configuration object with a few properties that rarely changes.
- High Memory Usage:
- Reason: The Memento Pattern involves storing multiple snapshots of an object’s state, which can lead to high memory consumption, especially if the object state is large or changes frequently.
- Example: An application that needs to maintain a large number of states (e.g., a complex graphics editor with numerous large images).
- Frequent State Changes:
- Reason: If the state of the object changes very frequently, managing and storing all those states can become impractical and inefficient.
- Example: A real-time gaming system where the state changes rapidly and requires constant updates.
- No Need for State Restoration:
- Reason: If there is no requirement for restoring previous states and you don’t need undo/redo functionality, the Memento Pattern may not be necessary.
- Example: A system where only the current state is relevant, and historical states are not needed.
- Security Concerns:
- Reason: If the state contains sensitive information, storing multiple snapshots of the state might pose security risks.
- Example: An application that deals with sensitive user data where storing multiple states might expose private information.